How to use React Native UUID
Request, Deliver, Revise, Done
Get unlimited UI Design & React Development for a fixed monthly price
50% off your first month
Introduction
If you want to generate a unique string to identify a particular piece of information, you’re going to want to reach for UUIDs. In most cases you’d generated your UUIDs on the server, but on occasion you might need to generate them in your React Native app. In this article I’ll show you how to easily generate UUIDs using the react-native-uuid package.
What are UUIDs?
UUID is short for Universally Unique Identifier (sometimes also known as a Globally Unique Identifier, or GUID).
UUIDs are 128 bit values that are commonly used for unique identification in software. There is a very tiny risk of collision - but it’s so low that you can essentially ignore it and treat the value as unique.
If you’ve ever come across a string that’s formatted like this:
ea9405f5-fc9f-49dd-872b-bf4f24b009d1
Then you’ve encountered a UUID.
Which UUID version?
In software development you are almost always going to be using UUID version 4, and for the purposes of this article that’s the version I’ll be referring to. However, just so that you’re aware, there are five versions of the UUID algorithm:
UUID Version 1
Version one joins together a timestamp and the MAC address of the device that’s generating the ID to create a value that’s unique to that device at that specific point in time.
UUID Version 2
This version is an evolution of version 1 for distributed computing environments that isn’t widely used.
UUID Version 3
Version 3 UUIDs are derived from two different pieces of input information - an input string and a namespace - and then converted into a UUID using the MD5 hashing algorithm.
UUID Version 4
This is the version that you will almost always encounter in software development. On the surface, v4 looks similar to v1, except that v4 is generated totally randomly, and contains no link to either the time of generation or the device it was generated on.
UUID Version 5
This is essentially the same as UUID version 3, except that it uses SHA-1 instead of MD5.
Installing React Native UUID
To install react-native-uuid, run this terminal command in your project root:
Or if you’re using Yarn instead of NPM, run this command instead:
Generating a UUID
To generate a UUID, first you need to import the uuid package at the top of your component file:
Then wherever you want to generate a UUID, simply call the V4 function on the UUID module, like so:
Complete example
Here’s a complete example of using react-native-uuid within a React Native component:
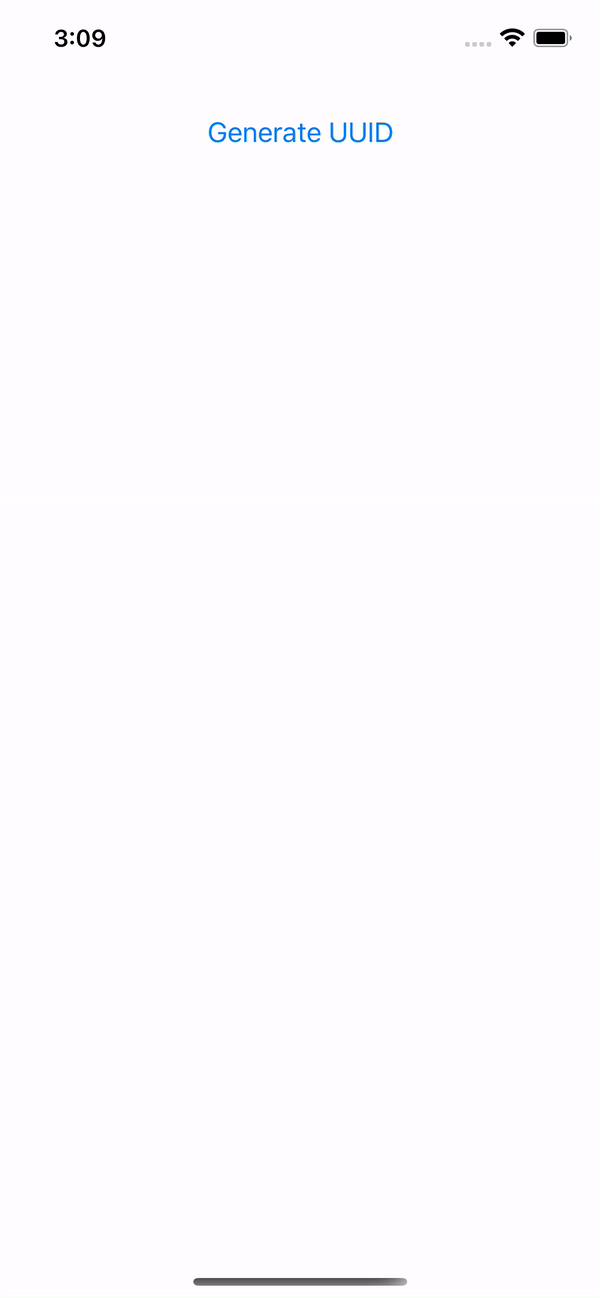