How to underline text in React Native
Request, Deliver, Revise, Done
Get unlimited UI Design & React Development for a fixed monthly price
50% off your first month
Introduction
When you have a block of text and you want to emphasise part of it, you'll usually want to use a text underline. In CSS you'd reach for the text-transform property, but it might not be immediately obvious how to do this in React Native.
In React Native there are three style properties that allow us to create and style an underline. In this article I'll show you some examples and how to use them.
Adding some text
To get started let's add a simple Text component with some lorem ipsum:
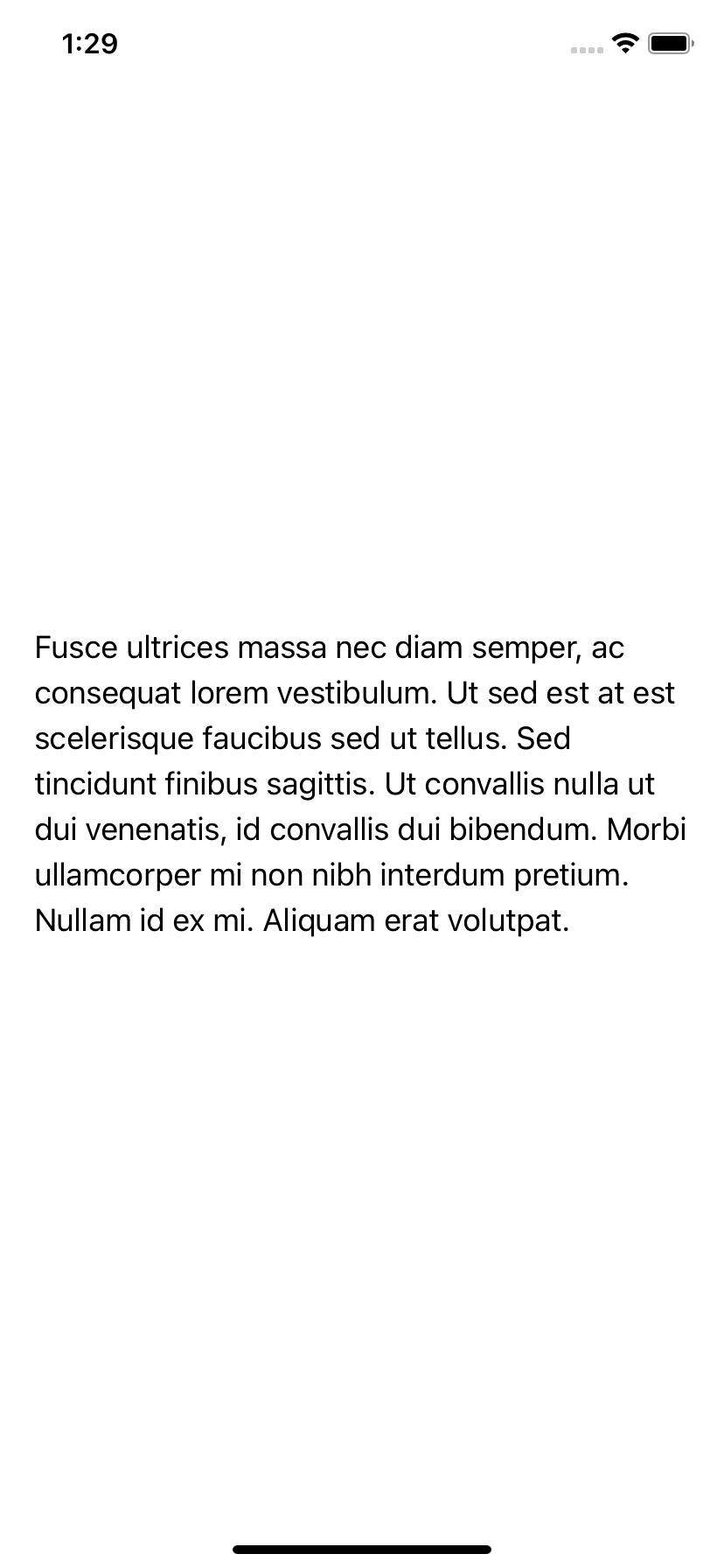
Adding a simple underline using the textDecorationLine property
We'll then add a nested Text component which we'll use to add our underline style to - the same way we'd use a span or a strong tag in HTML.
To add our underline style, we use the textDecorationLine property, and set the value to 'underline':
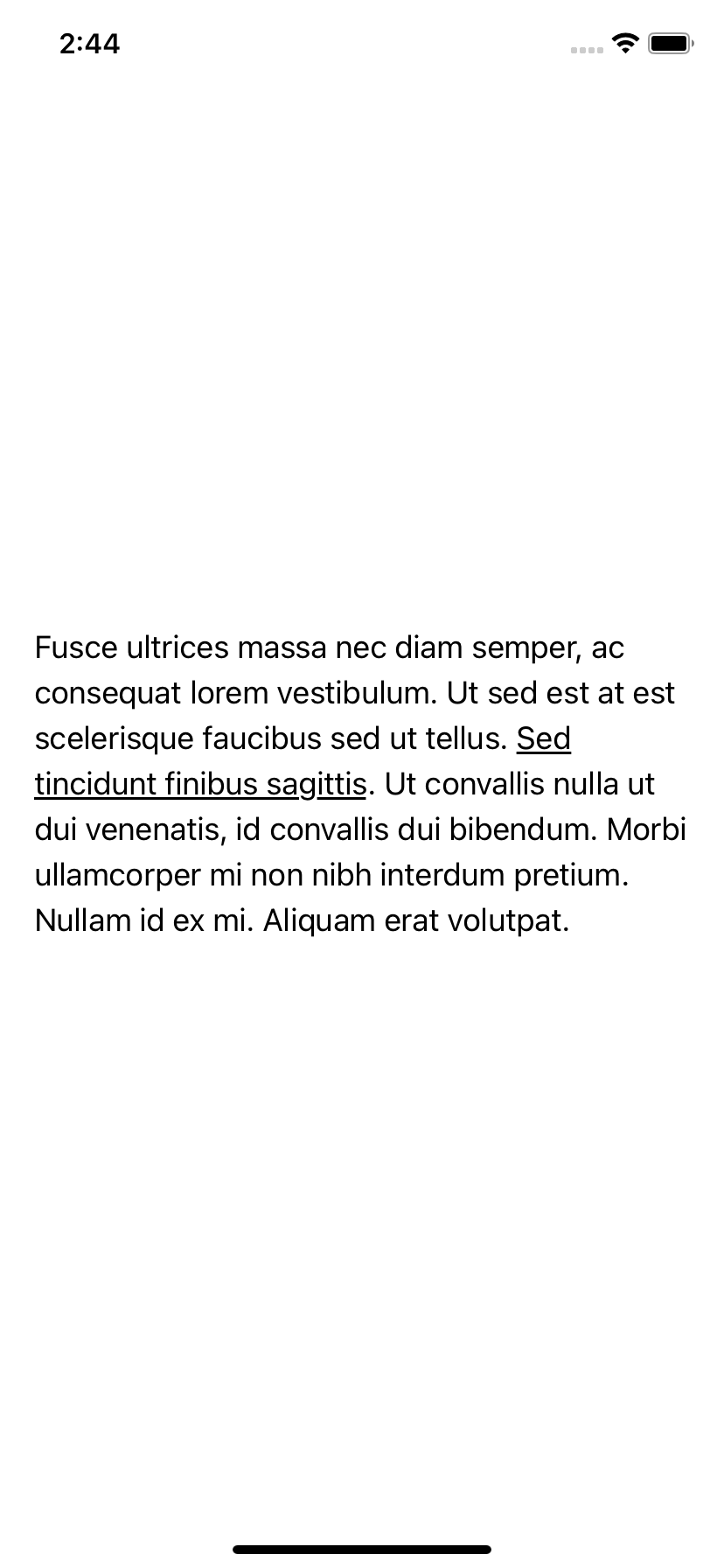
Adding a color
If we want to add a color to our underline, we need to add the textDecorationColor property and set it to the value of the color we want to use:
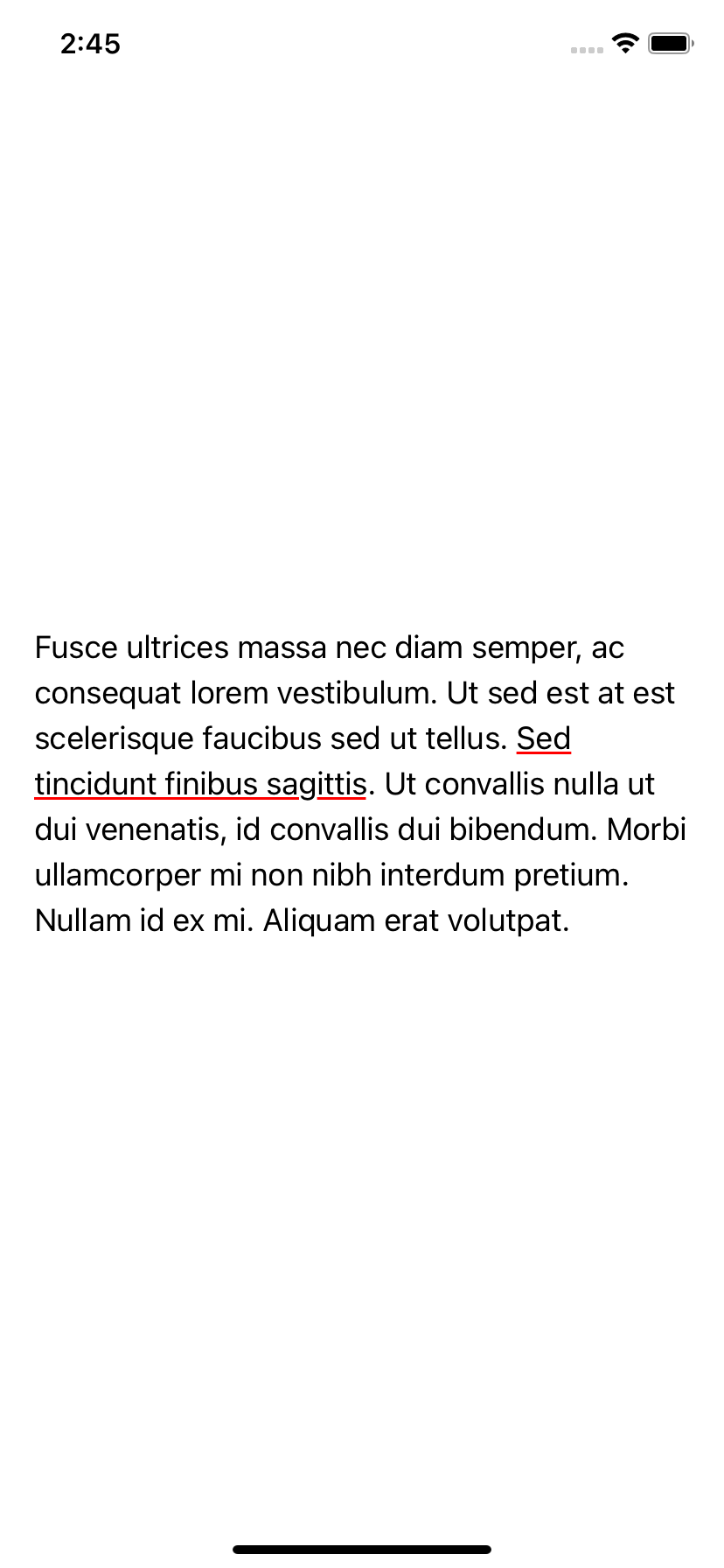
TextDecorationStyle dotted
You can also change the type of underline, just like you can in CSS, using the textDecorationStyle property. For example, if we want to add a dotted underline, we set textDecorationStyle to 'dotted':
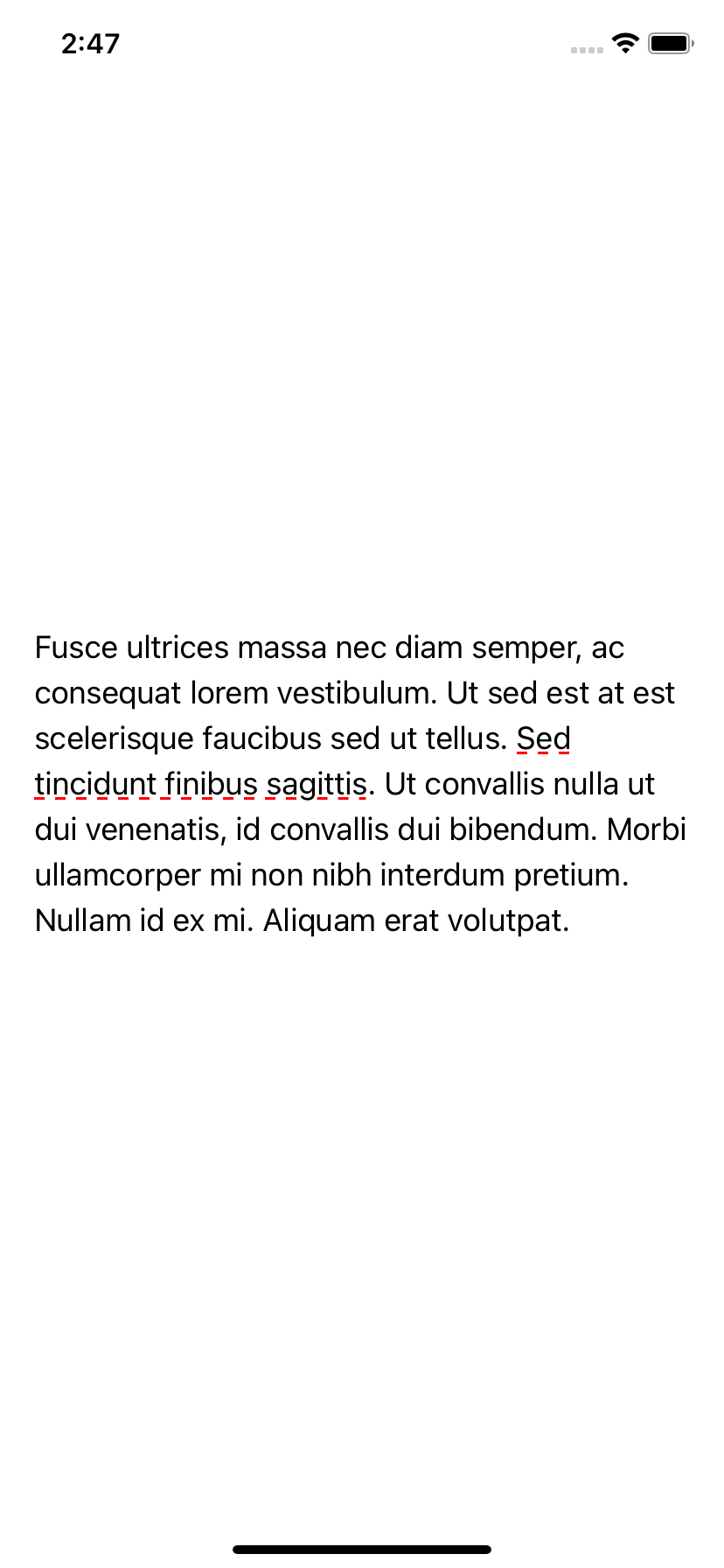
Dashed
If you want to add a dashed underline, set textDecorationStyle to 'dashed':
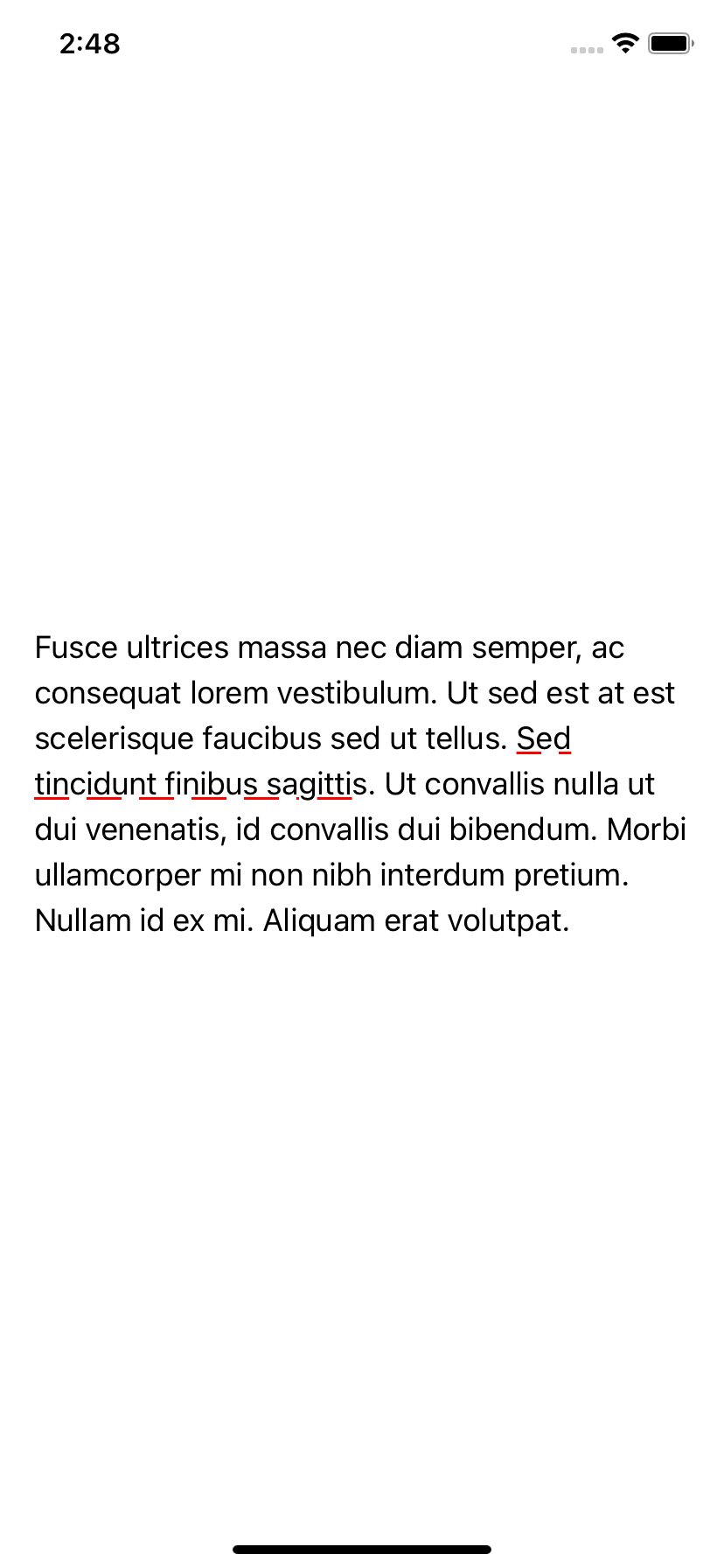
Double
To add a double underline, set textDecorationStyle to 'double':
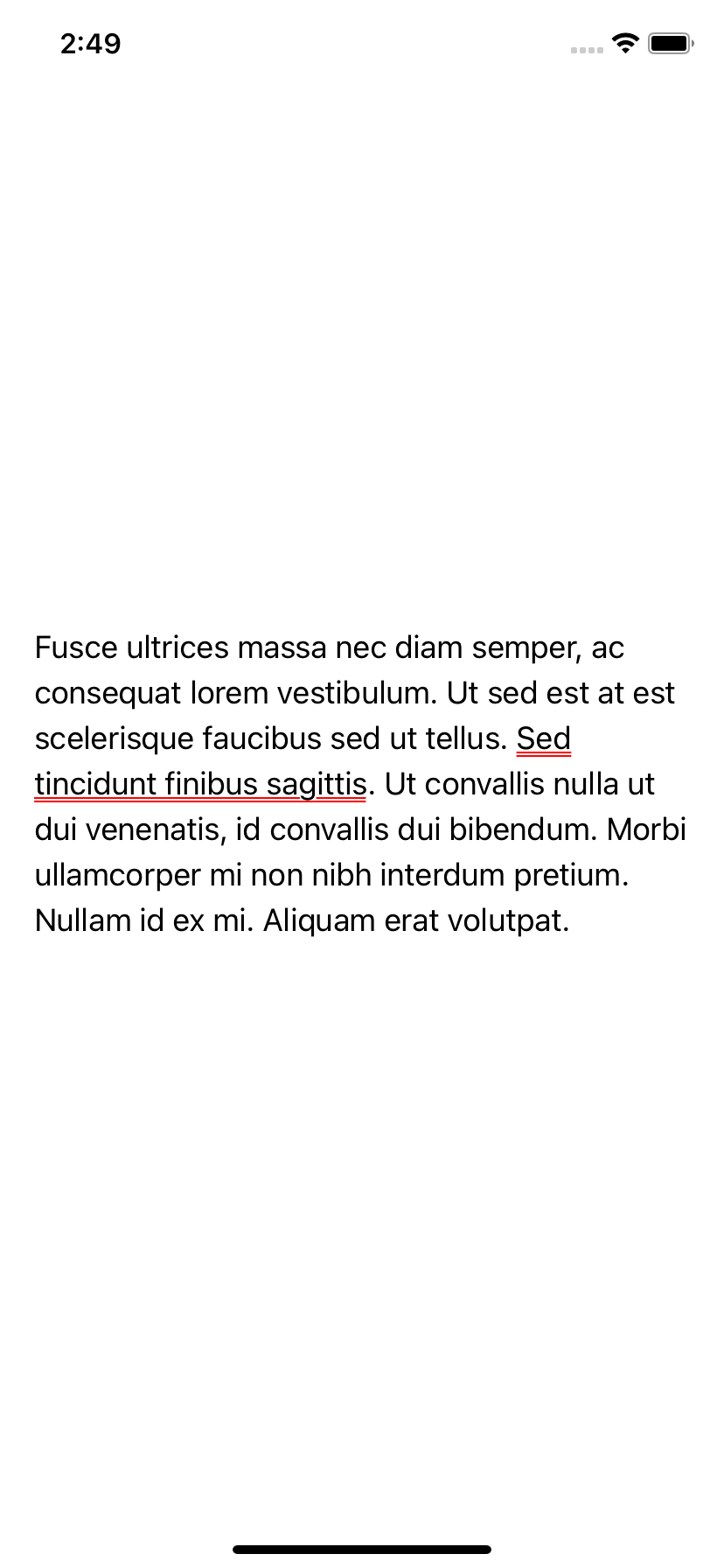
More Information
For a full list of available Text style props, you can check out the React Native documentation page.