How to build a star rating component with React Native ⭐️⭐️⭐️⭐️⭐️
Request, Deliver, Revise, Done
Get unlimited UI Design & React Development for a fixed monthly price
50% off your first month
Introduction
Building a movie listings app? Or an airbnb style real estate rental app? There are many situations where you’ll need to add user ratings to a React Native project. When this happens you’ll likely want to build a simple star rating component.
A note on icons
For this tutorial we’re using Expo Vector Icons for our star icons, which ships automatically with projects created via Expo’s CLI. If you’re using a non-Expo React Native project, you can use React Native Vector Icons as a direct replacement.
Of course, feel free to use whichever icon library you like instead! Those are just the ones I’ll be using in this tutorial.
Scaffolding our star rating component
Let’s set up the basic structure of our app. First we’ll create a SafeAreaView to deal with device notches, and a View that will act as our container:
Now we’ll add our five stars using the MaterialIcons component from expo vector icons, as well as a simple heading:
Now we have the basic structure of our star rating component:
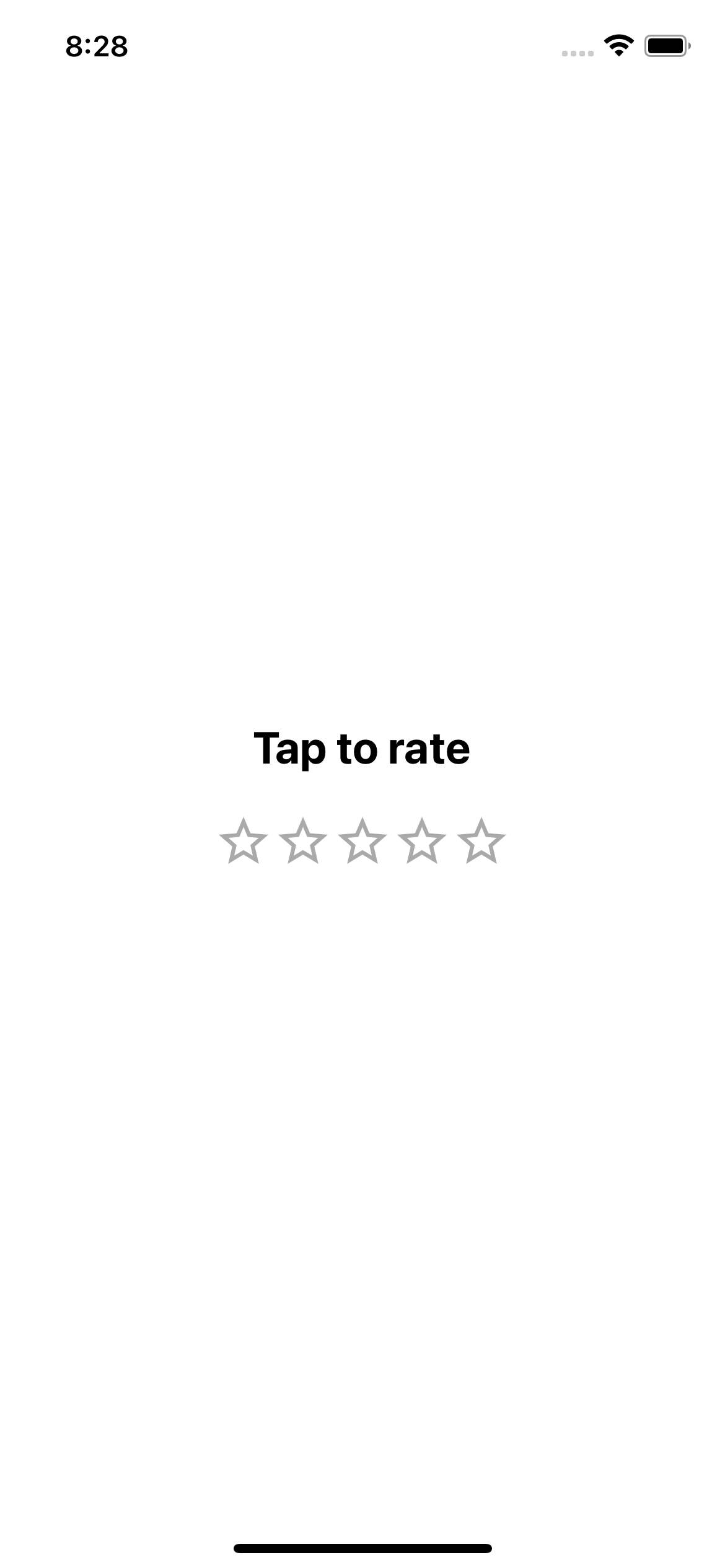
Adding star rating with useState hook
Now we need to add a state value that will store our rating to make it interactive. Lets import the useState hook from react and create a starRating state value:
Now for each of our stars we’ll add an onPress function that will set the starRating value. We’ll also set a ternary operator in our heading to display our star rating if selected, and an instruction if not:
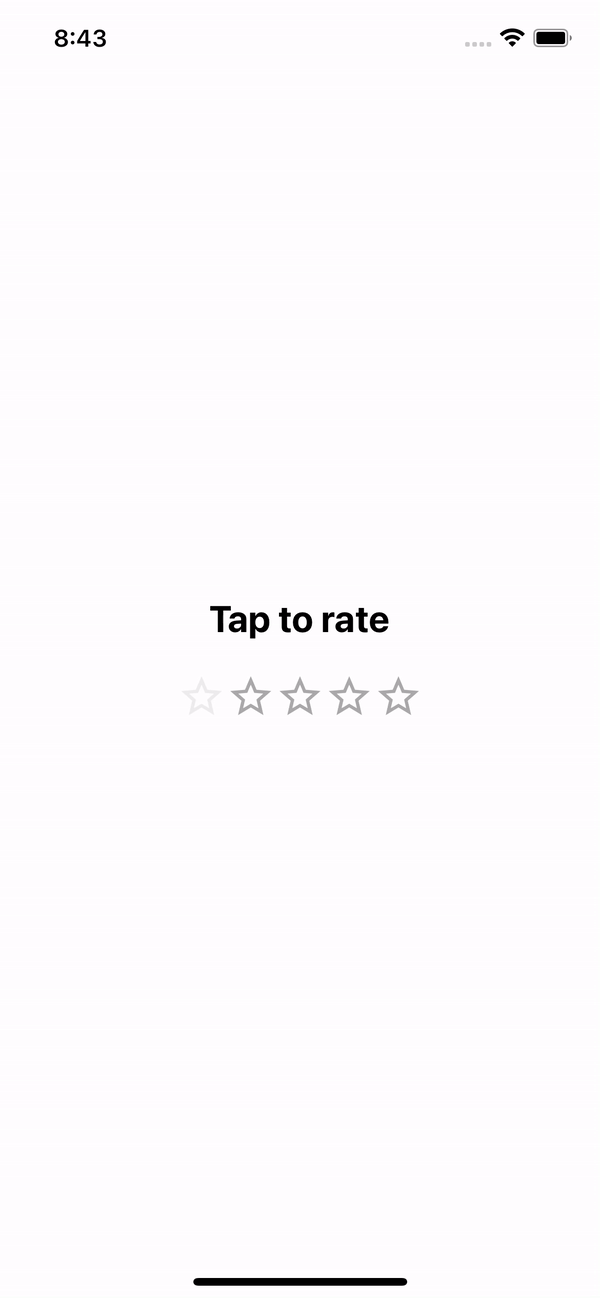
Styling our star rating component
Now we can set a star rating, let’s style our star rating component. For each of our stars we’ll add a ternary operator to the style property that will check if the star’s value is equal or greater than the current starRating value - if it is, it will use the starUnselected class, if it isn’t, it will use the starSelected class which we’ve added below:
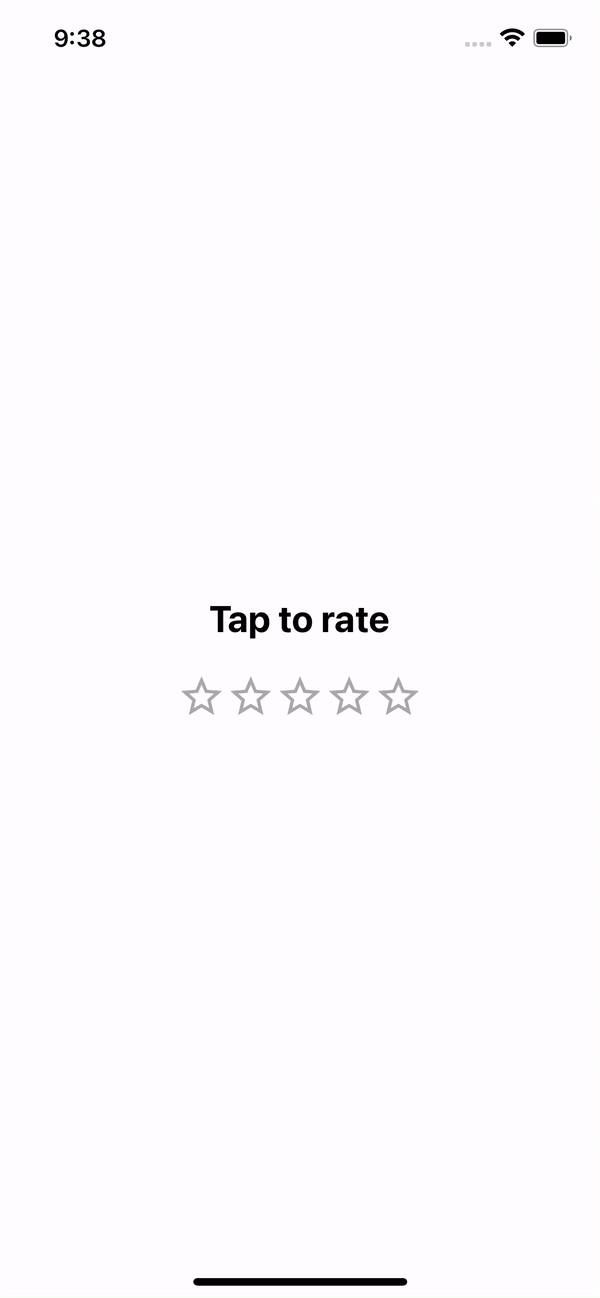
Adding animation with the Animated API
We now have a working star rating component, but the actual animation looks a bit barebones. Let’s use the React Native Animated API to give some better visual feedback to the user.
First we need to switch out our TouchableOpacity wrappers to TouchableWithoutFeedback instead:
Now we add onPressIn and onPressOut functions that wlll scale our stars using a transform value:
Here’s our updated star rating component with our improved animation!
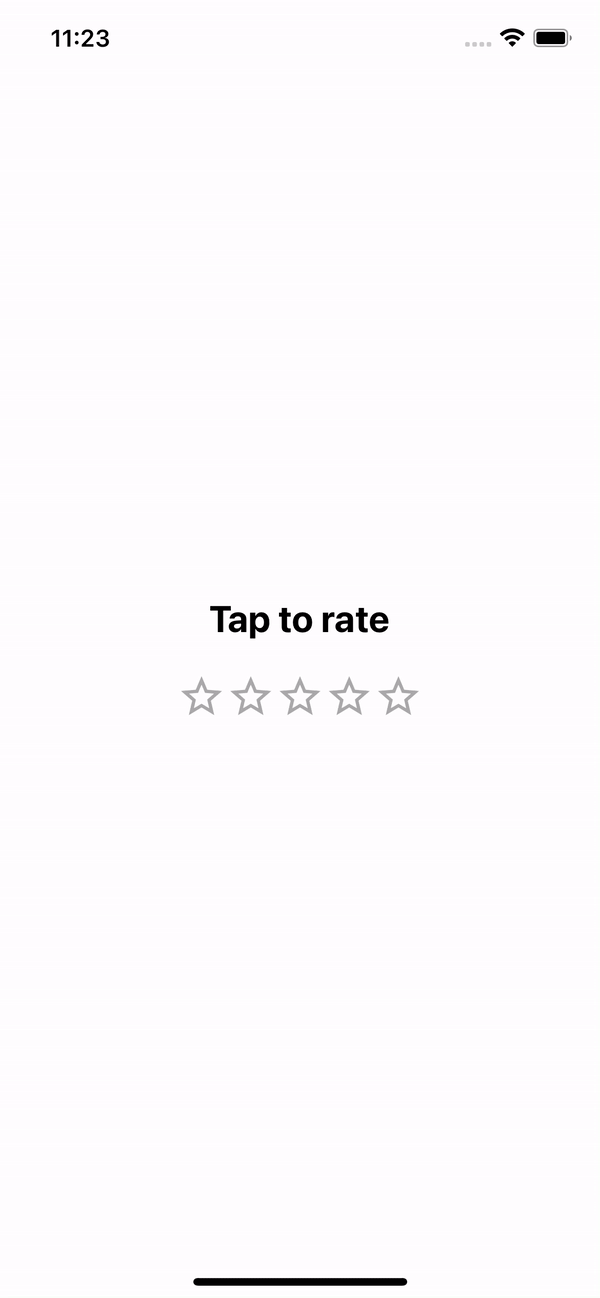
Using an array for our star rating options
If you want to make the component a little tidier, you can use an array to store and loop over the star rating values. Another advantage of this is that you can easily change the star rating scale from 5 to whatever you like:
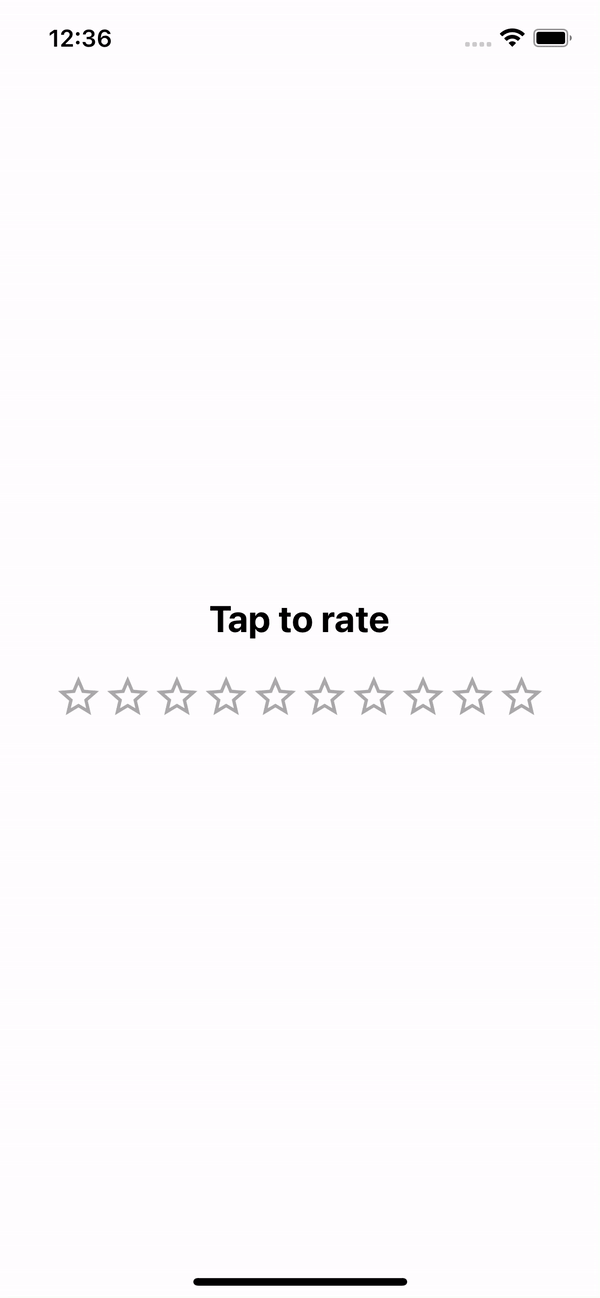