How to create rounded corners in React Native
Request, Deliver, Revise, Done
Get unlimited UI Design & React Development for a fixed monthly price
50% off your first month
Introduction
With modern UI design, rounded corners have become ubiquitous in mobile apps and you may be wondering how to achieve the same effect in React Native. Luckily it’s very easy to achieve using a property called border radius (something you’ll be familiar with if you’ve ever written CSS). Read on to find out how!
Why use rounded corners?
You may have noticed that rounded corners are used very widely in modern UI design. Go to the website or app of any big startup and you’ll likely find that rounded corners are widely used.
Quite simply, rounded corners are in general more appealing to the human eye. There are many theories as to why this is - but the most enticing in my opinion is that you are far more likely to encounter rounded corners in nature (when was the last time you saw a square in the woods?).
We also learn to avoid sharp edges from a very young age because they’re dangerous. Therefore, it’s not a stretch to think that rounded corners in user interfaces naturally give us a feeling of safety and trust.
Another thing to consider is that rounded corners, by their nature, place the focus of the users eye on the content of the rectangle. Whereas sharp edges tend to draw the users eye away from the content. Thus rounded corners make it easier for the user to quickly scan the content on the page and to group items visually.
How to use border radius in React Native to create rounded corners
It’s very, very easy to create rounded corners in React Native. To demonstrate, we’re going to create a very simple button. To get started, import both the TouchableOpacity and Text elements from React Native at the top of your component file. We’ll also import SafeAreaView which will act as our container:
Let’s add a SafeAreaView container that we’ll use to centre our button:
Now let’s add our button - we’ll make it blue and give it a padding of 10. We’ll also add our text inside of it and make it white:
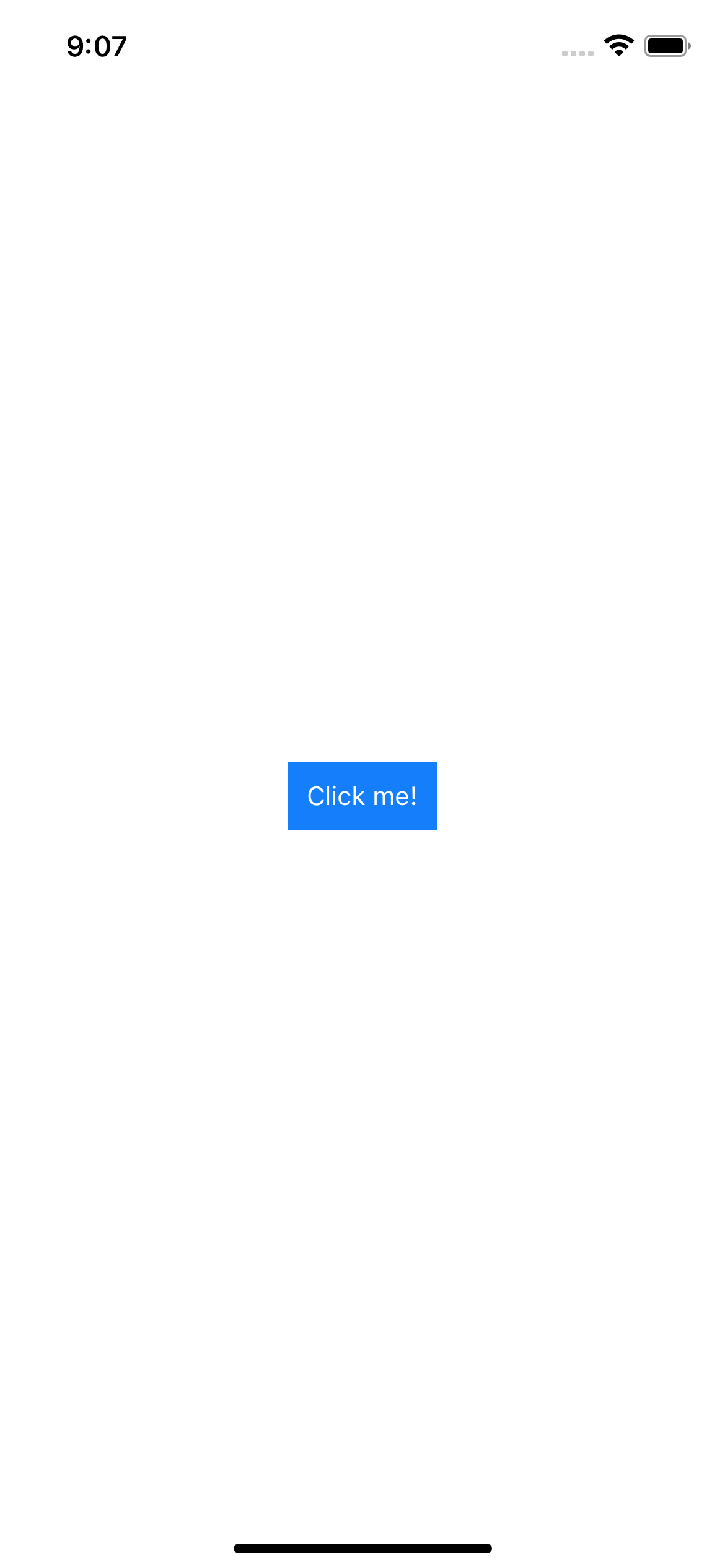
That gives us a square button. In order to add rounded corners, all we need to do is add a borderRadius property to the style of our TouchableOpacity, and give it whatever radius value we desire (I’m going to make it 4 in this example):
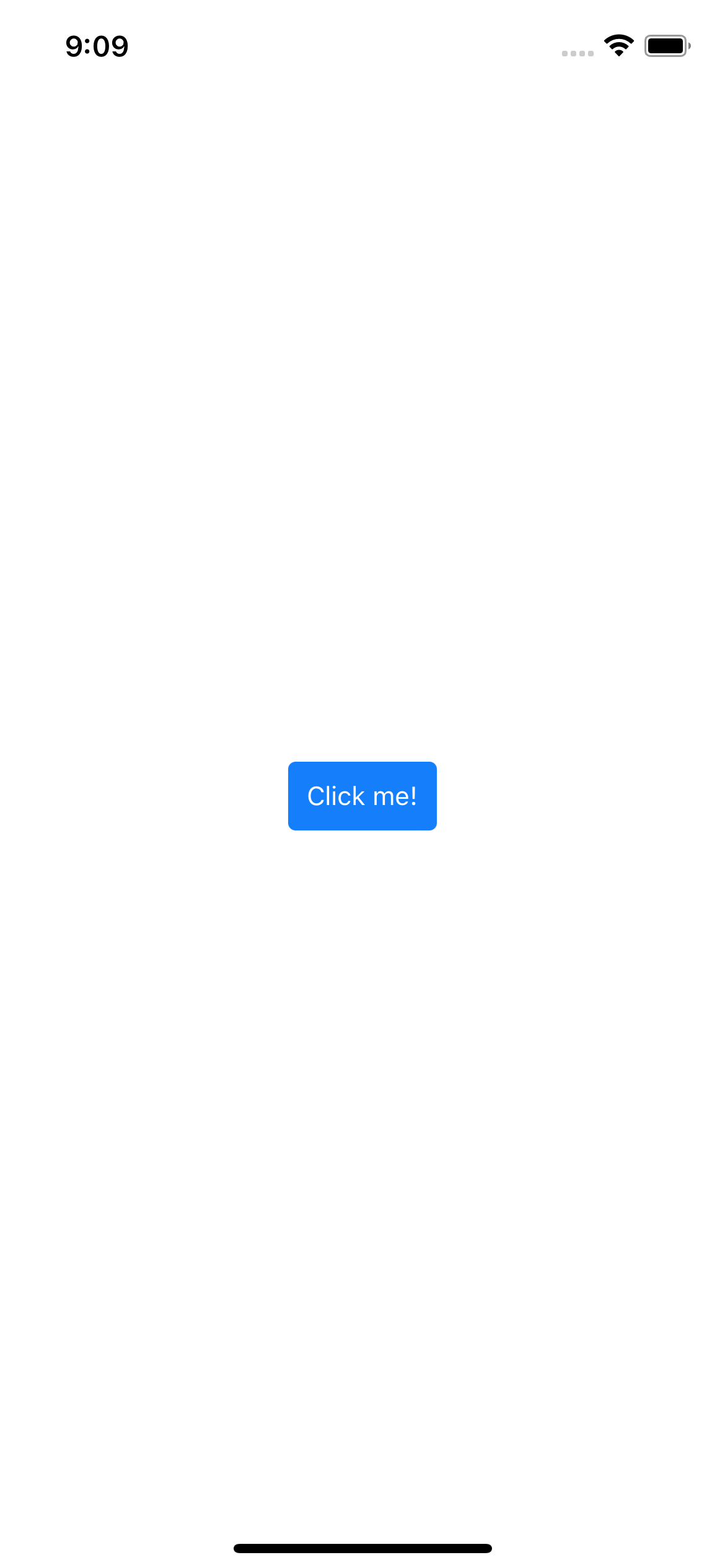
It’s as easy as that! Now we have rounded corners on all sides - but what if we wanted to only give individual corners a border radius? We can do that using the borderTopLeftRadius, * borderTopRightRadius, borderBottomLeftRadius and borderBottomRightRadius properties.
For example, if we wanted to just make the top left and bottom right corners rounded, we’d set the style as follows:
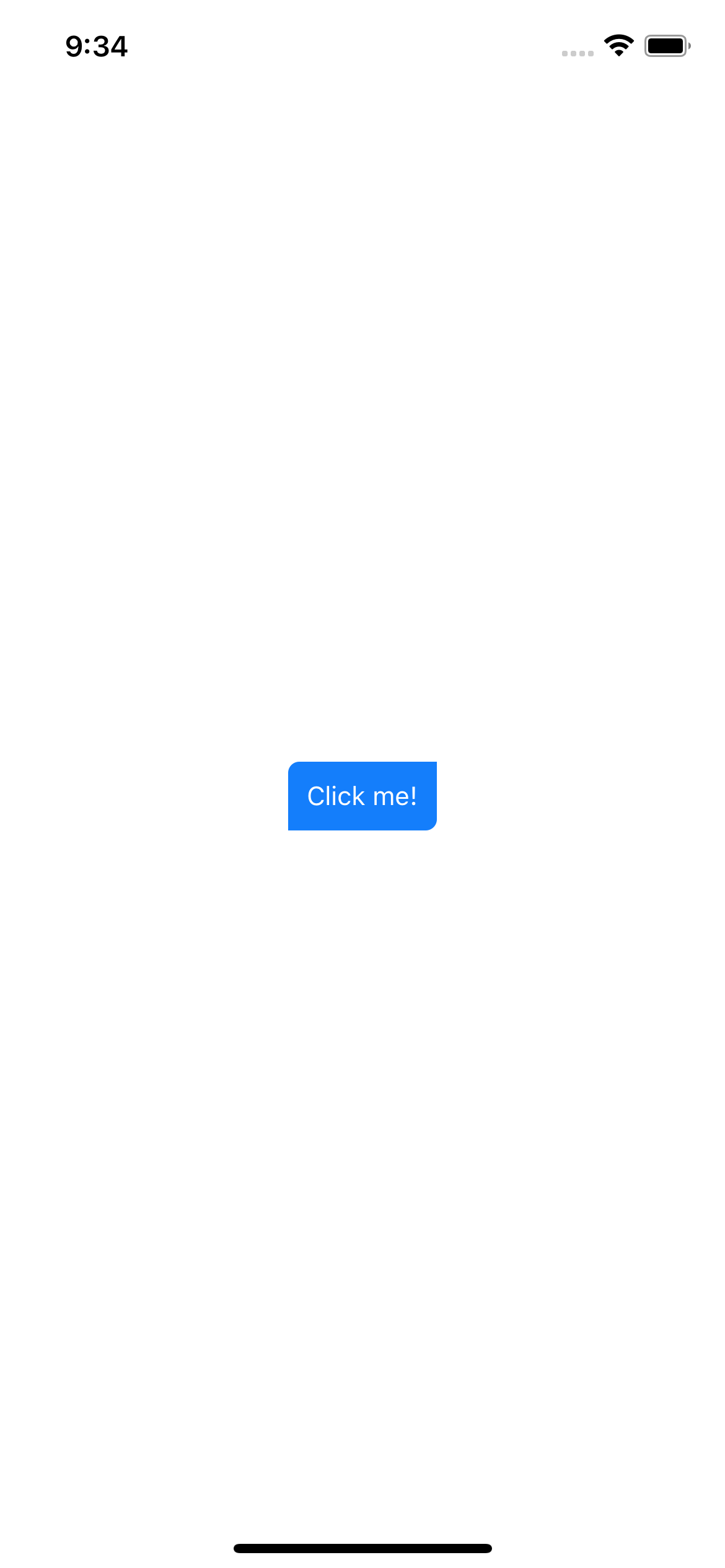
And that gives us a border radius on just those individual corners.
Full code example
For your reference, here is the full code example:
Summary
There you have it - as you can see creating rounded corners is ridiculously easy in React Native using the border radius property. I hope you found that useful!