A guide to platform select and platform specific files in React Native
Request, Deliver, Revise, Done
Get unlimited UI Design & React Development for a fixed monthly price
50% off your first month
Introduction
React Native is designed to work across multiple platforms seamlessly, without having to write code multiple times - and for the most part, it does that. It’s pretty rare that you’ll actually need to write some logic specifically for iOS, Android or the web (or Windows or MacOS nowadays). But when you do, you’ll need to use either platform specific files or the platform select method.
What are platform specific files?
The easiest way to write platform specific code is to simply use platform specific files. React Native allows you to add an extension which will override a component for a particular operating system. This is incredibly handy if you just want to override and entire component (if you’d prefer to just override something within a component, see the platform select section below).
How to use platform specific files in React Native
Let’s say you have a file called Calendar.js. If you want to create an iOS specific version of that component, all you need to do is create another file called Calendar.ios.js - you simply write your iOS specific version of the component in that file and React Native will automatically use that version when running your app on an iOS device. On all other non-iOS devices, it will use Calendar.js as normal.
Here are the platform specific file extensions you can use:
.native - this will target both iOS and Android .ios - this will target iOS devices .android - this will target Android devices .web - targets the web version when using React Native Web .macOS - targets MacOS devices when using React Native MacOS .windows - targets Windows devices when using React Native Windows
What is platform select in React Native?
Platform.select is a method on the Platform module . It allows you to write platform specific logic within your component files, without having to write separate files.
Platform.select takes an object where the keys are the different devices that we want to target. Depending on which device the user is running, it will return the most appropriate method.
How to use platform select in React Native
In order to use Platform.select, simply pass it an object with keys of the the different devices you want to target:
In this example, a different text value will be returned by the textValue function depending on what type of device the user is running. If they’re running an iOS device it will return the value of the iOS key, if Android it will return the value of the android key, and any other device will return the value of the default key. Here is what it looks like on iOS and Android:
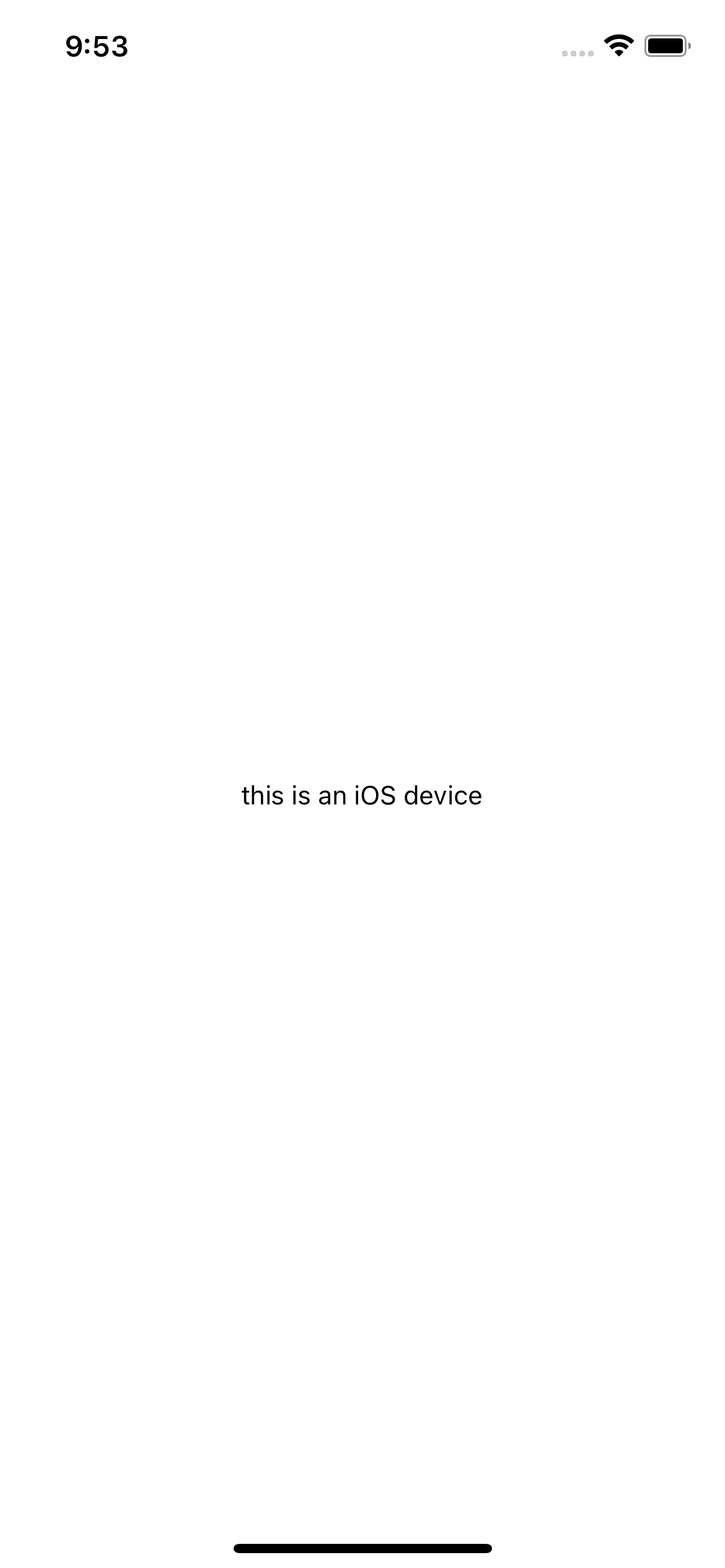
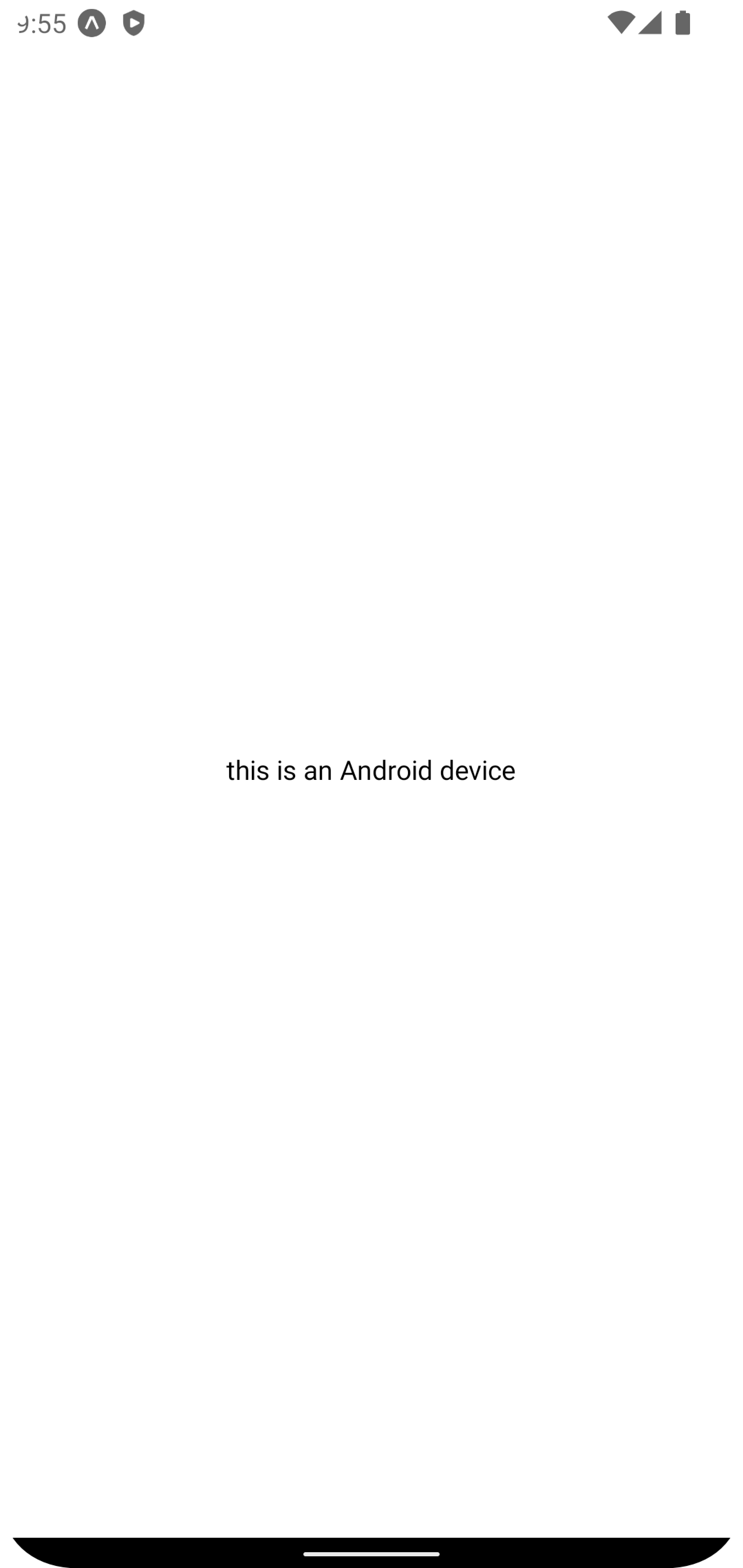
You can also use Platform.select within the StyleSheet module to create platform specific styles. Here’s an example:
As you can see this returns a different text colour depending on the current device:
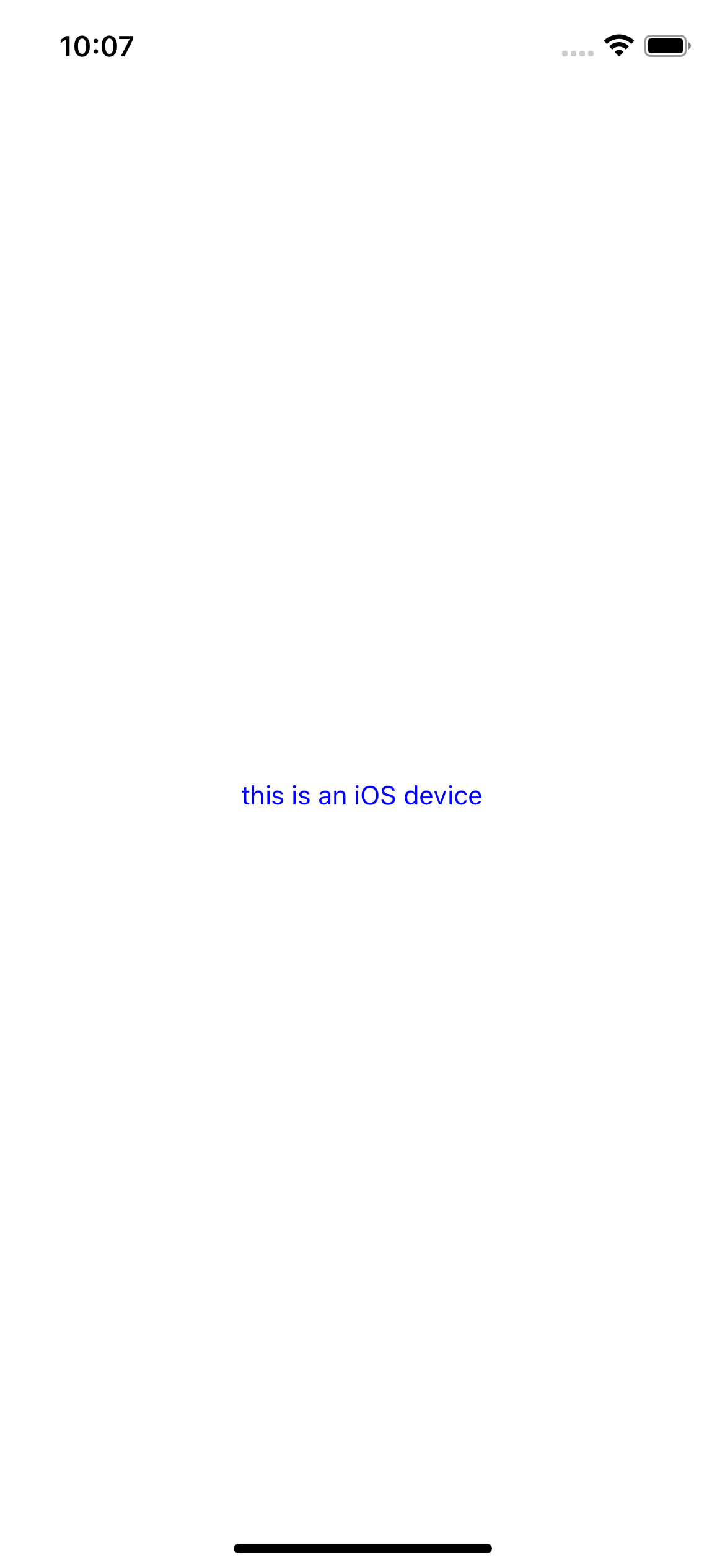
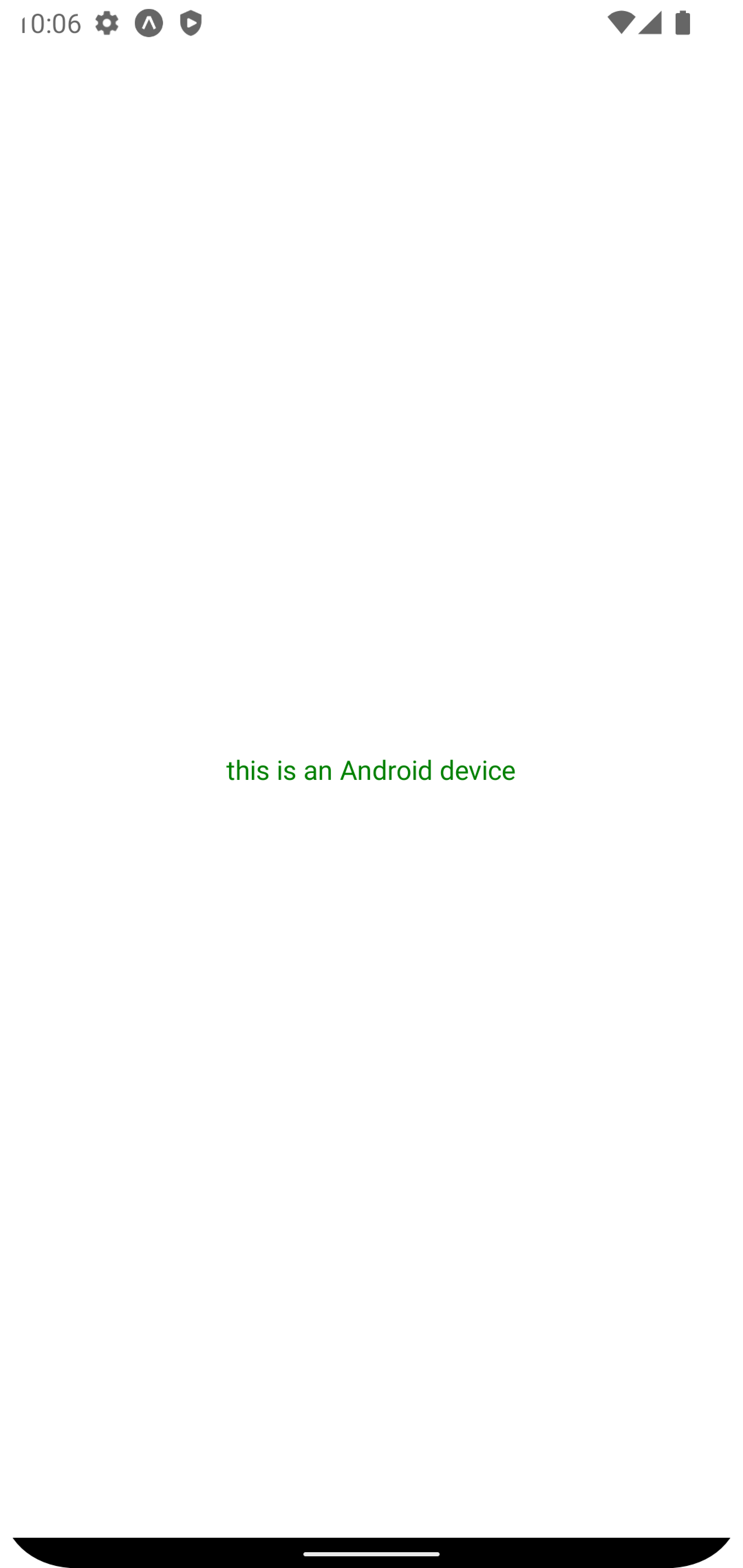
Using the Platform.OS method to return the type of device
If you simply want to return the name of the current OS, you can do so using the Platform.OS method:
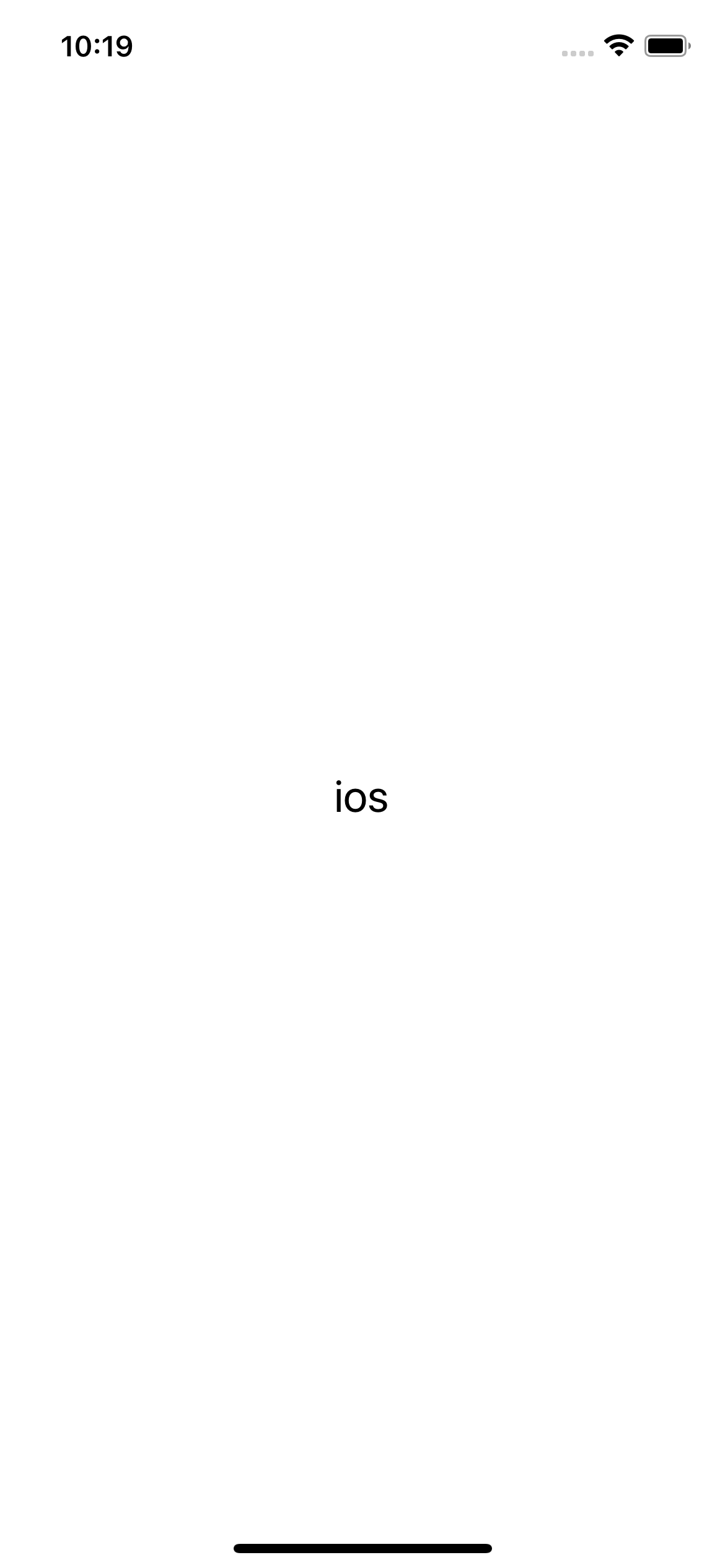
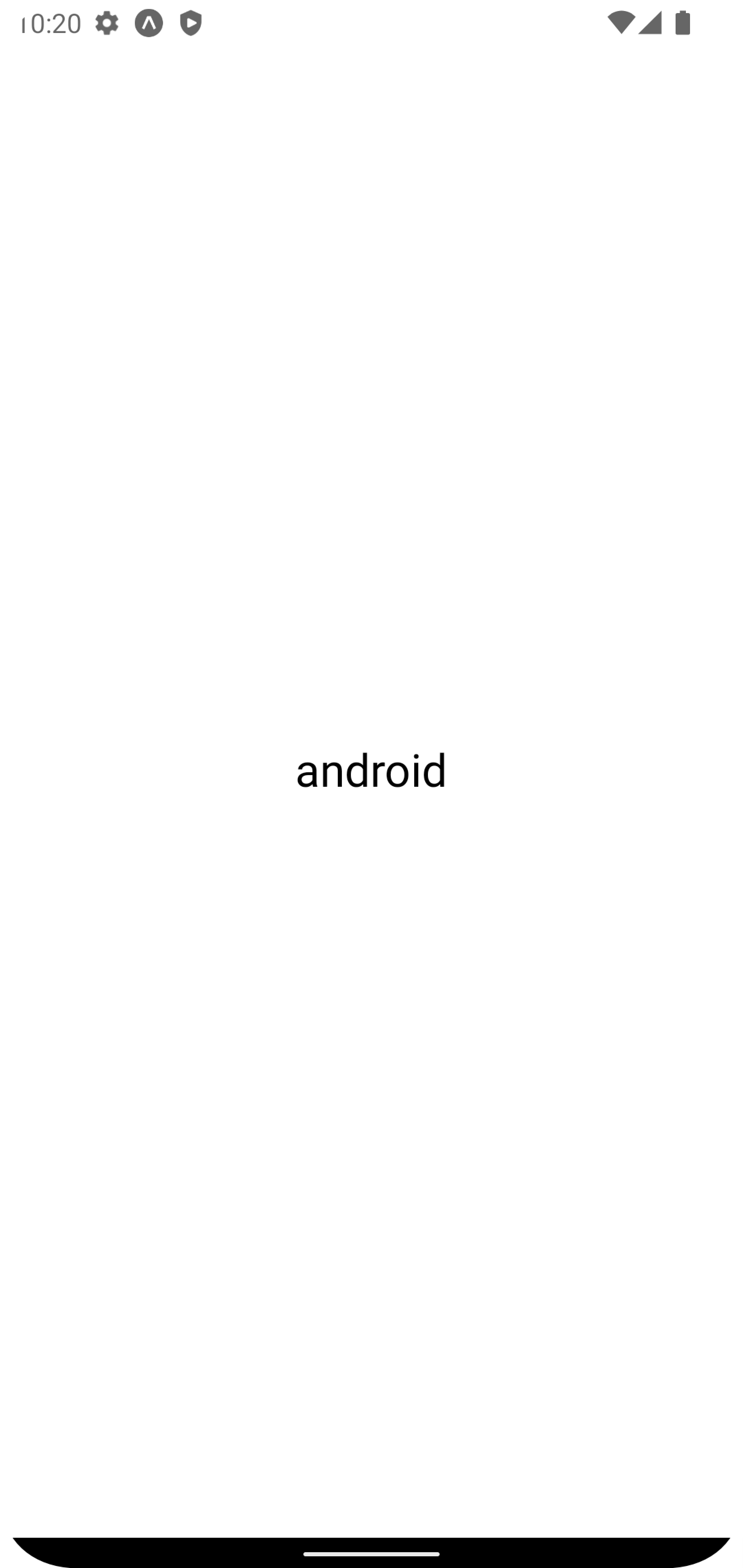
Summary
So there you have it, for those occasions where you need to write some platform specific code, there are three easy options you can use. I tend to use a mixture of all three methods in my projects.