How to open a link in the browser from a React Native app
Request, Deliver, Revise, Done
Get unlimited UI Design & React Development for a fixed monthly price
50% off your first month
Introduction
You might occasionally need to link to an external website from inside your React Native app. In this article I’ll be covering how you open a link in the users default browser using the React Native Linking API.
What is the React Native Linking API?
The React Native Linking API is an interface that allows you to handle both incoming and outgoing links in your app. In addition to HTTPS links for the web, you can also use it to handle outgoing email, telephone and SMS links from your app.
For example, if you wanted to give your customers an easy to way to send you an email, you would create a mailto link from your app using the Linking API and when the user tapped it it would automatically open their preferred email client with the to field of the email already filled out.
You can also use it to handle deep linking for incoming links - where the user has opened your app via an external link using a custom scheme.
But for today’s article, I’m just going to show you probably the most simple use case - opening a link from inside your app that will open in the users preferred browser.
Opening a link in the browser using React Native Link
First things first, let’s create a very basic scaffolding for the component that will contain our external link:
Let’s add the Linking module to our React Native imports, along with TouchableOpacity which we’ll use to actually create the link:
Now in our component we’ll add a TouchableOpacity that will open a link to Google using the onPress method. All we need to do is call the openURL function on the Linking module and pass in our URL (remember to include the https prefix):
And voila! Our link now opens in the users browser.
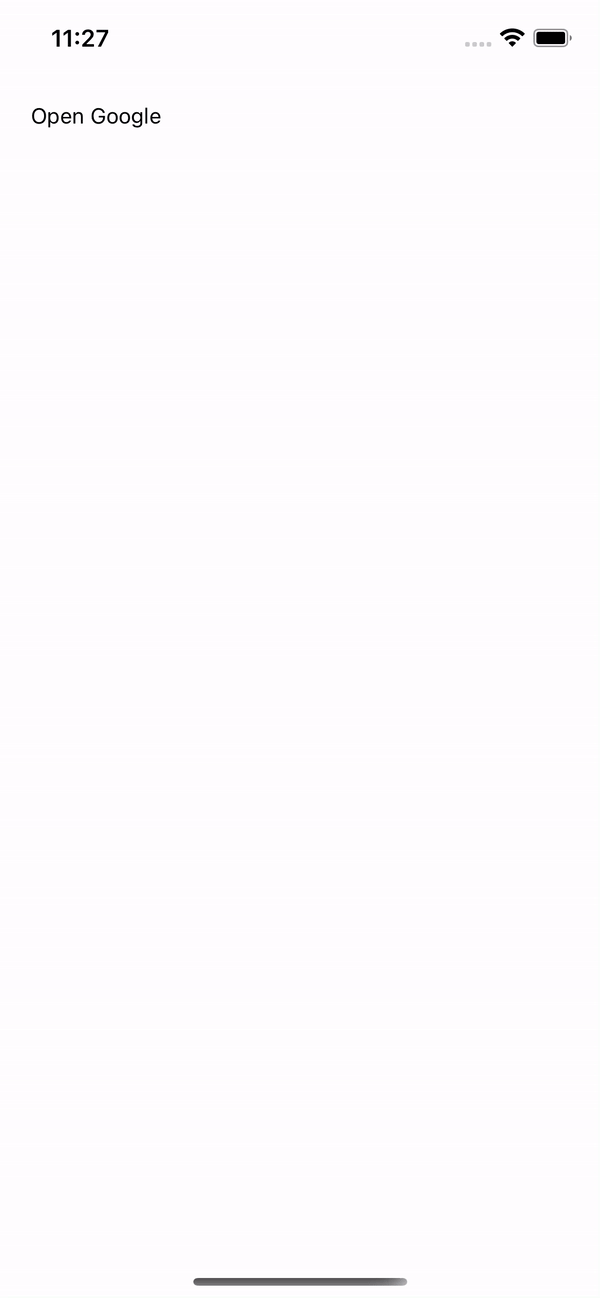
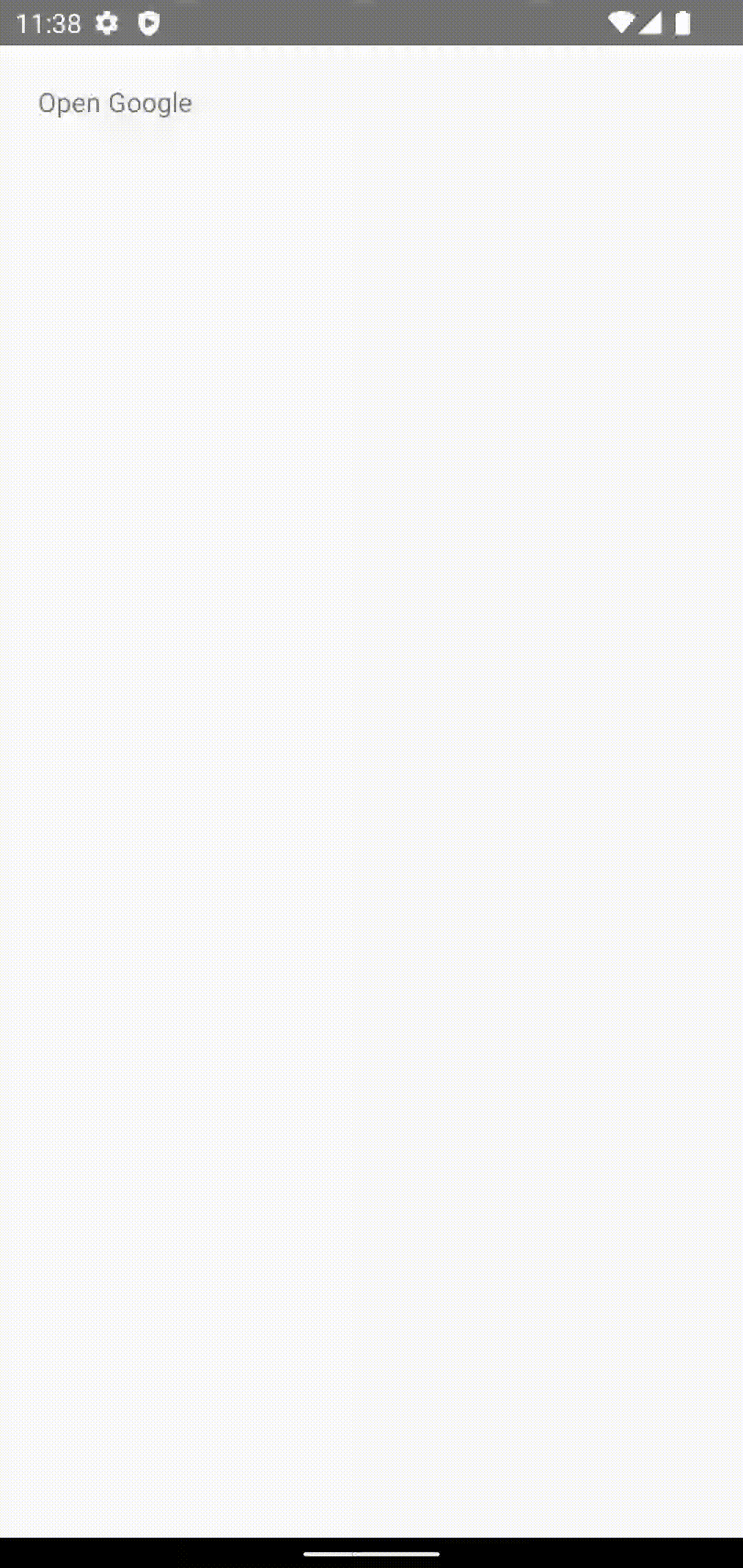
Full example code
See below for the full example code:
Does Expo support the React Native Linking API?
Expo does not support the React Native Linking API - instead it implements its own linking package. I haven’t used it myself, but for the purposes of this particular tutorial you can just use the Expo module as a direct replacement as the openURL function works exactly the same in both packages.
Summary
So there you have it, you can now open a link in the browser from a React Native app. It’s a relatively simple bit of functionality but one you’re likely to run into during your React Native journey. I hope that was helpful!