React Native display none equivalent - how to hide an element
Request, Deliver, Revise, Done
Get unlimited UI Design & React Development for a fixed monthly price
50% off your first month
Introduction
If you're coming to React Native from web development, you're probably used to hiding an element by setting the display property to none in CSS - and you might be wondering if there's an equivalent method in React Native. The answer is… sort of.
You can show and hide an element in React Native - but the way you'd approach it is slightly different than in HTML and CSS. In this article I'll show you how to do it.
What is display none in CSS?
If you're not familiar with CSS, using display none is the most common way most people would hide an element. So if your element had a class of hidden-element, you'd set the display property on that class to be ‘none', and the user wouldn't be able to see the element in their browser:
Is there a display none equivalent in React Native?
There is no direct equivalent of display none in React Native - this is because React Native shows and hides elements based on your application state. So rather than setting an elements visibility directly, you would show or hide an element based on a state value.
Showing and hiding an element in React Native with useState
Let's use a basic example to show how you'd achieve this with React Native. First we'll create a boolean state value called elementVisible using useState that will decide whether to show our element.
First, we'll import the useState hook from React at the top of our component file:
Then in our component we'll declare a new state value called elementVisible, setting it initially to be false:
Now let's create a container for our element. We'll need to wrap it in a SafeAreaView so that our app accounts for device notches. We'll also nest a View element that will center it's content using flex:
Then we'll add our element with some basic styling. We'll also add a button that we'll use to toggle the visibility of our element:
In order to change our state value, we need to add an onPress function to our button. In the onPress function we'll call the setElementVisible function, and set it to the opposite of the current elementVisible value. As the value is initially set to false, our function will set it to true when the button is first pressed:
We'll then wrap our element in a ternary operator, which will only show it if elementVisible is set to true:
We'll also use a ternary to set the title of our button based on whether the element is visible or not:
And voila! We can now show and hide our element (keep scrolling for the full code example):
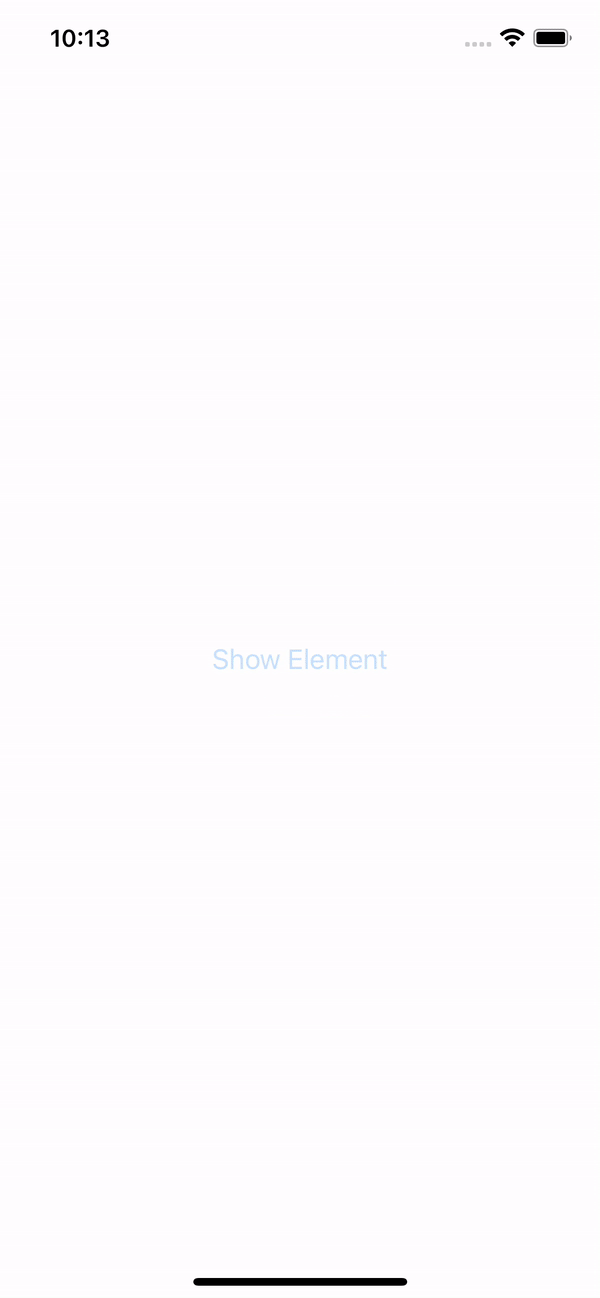
Full component code
Here's the full code for our show and hide example: