An easy way to create a bullet list in React Native
Request, Deliver, Revise, Done
Get unlimited UI Design & React Development for a fixed monthly price
50% off your first month
Introduction
Something you will commonly find yourself needing to do in React Native is create a bulleted list, but it isn't as straightforward as you might think. Unlike in HTML and CSS, there is no unordered list tag that will add the bullets for us - instead we need to use unicode to add them.
For code examples you can simply copy and paste, built with Tailwind CSS - check out our List components page.
How to create a bulleted list in React Native with unicode
First, let's use the FlatList component to create a simple list that we can then style. Here is the basic structure of our component:
In our renderItem component, let's add some unicode to create a bullet effect. We're going to use the u2022 unicode characer which is a simple bullet icon. We use javascript template literals to insert it into our text component alongside whatever value we want to display:
Note: remember to add a backslash in front of your unicode entity, otherwise it will just print the id!
Now we have our very simple bullet list:
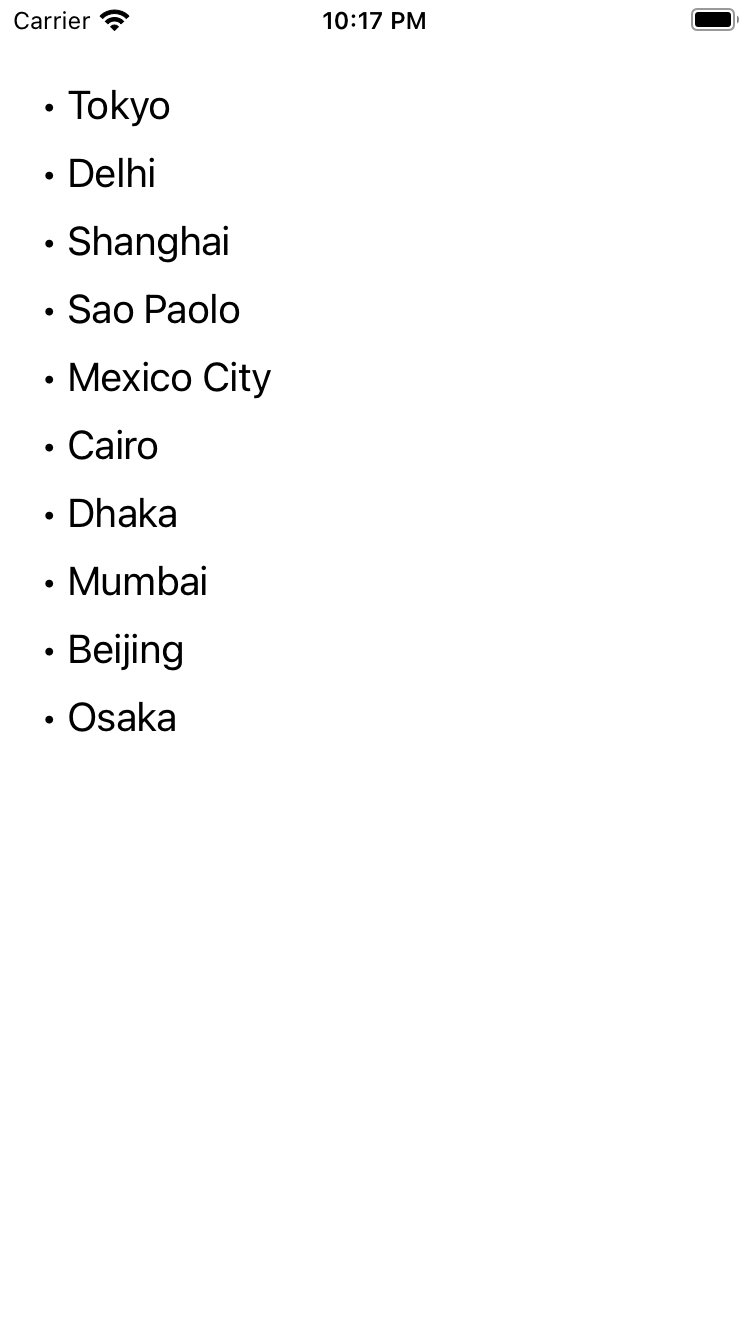
If you want to use larger bullet points, you can use the u25CF character instead:
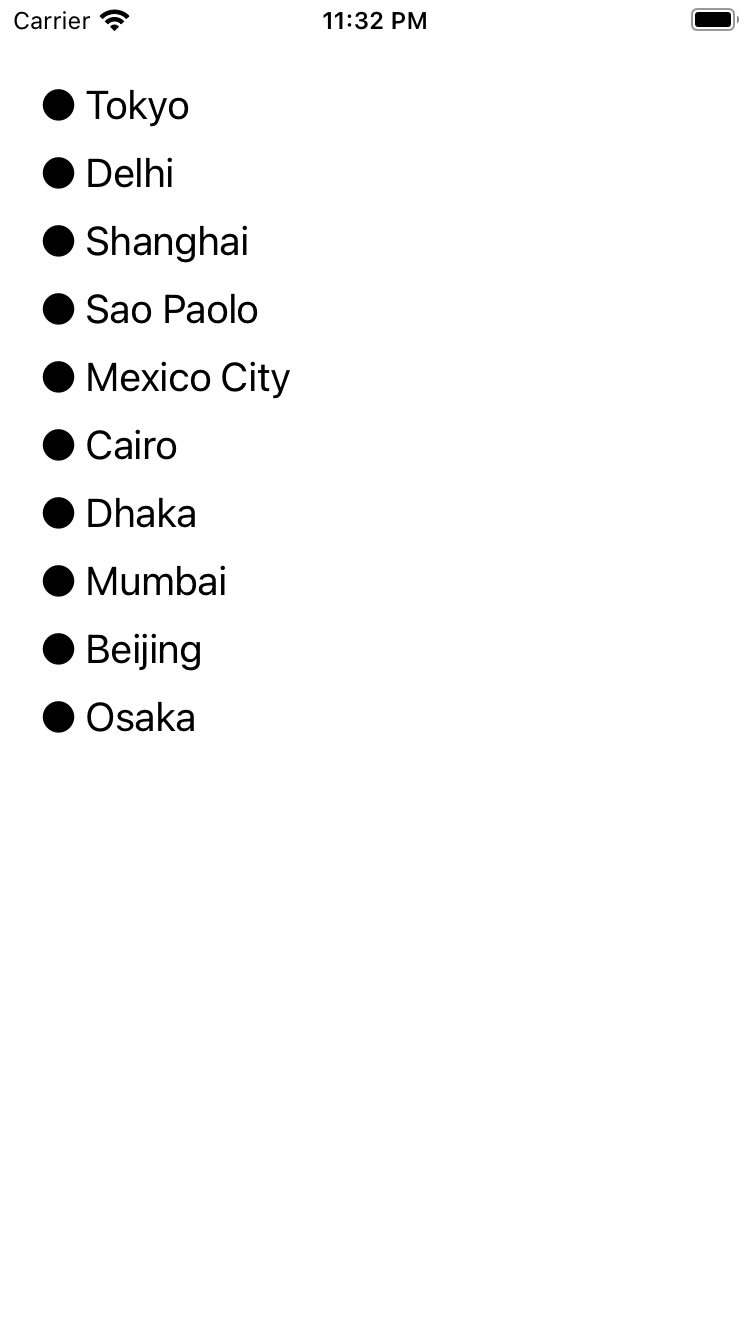
These work for most use cases - but you can use whatever unicode entity you want to style your bullet list however you like. Keep reading for some other examples.
How to create a hyphenated bullet list in React Native
You can use a hyphenated bullet by using the u2043 unicode:
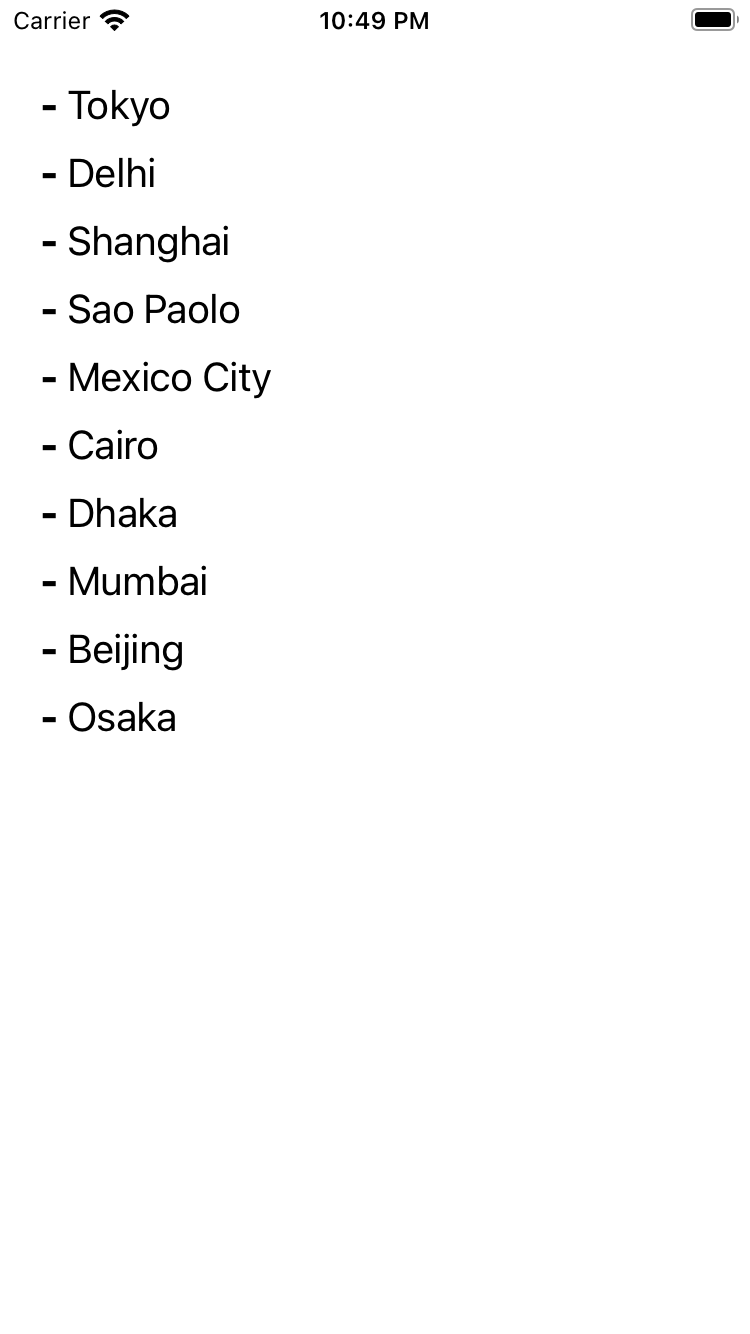
How to create a square bullet list in React Native
You can use a hyphenated bullet by using the u25AA unicode:
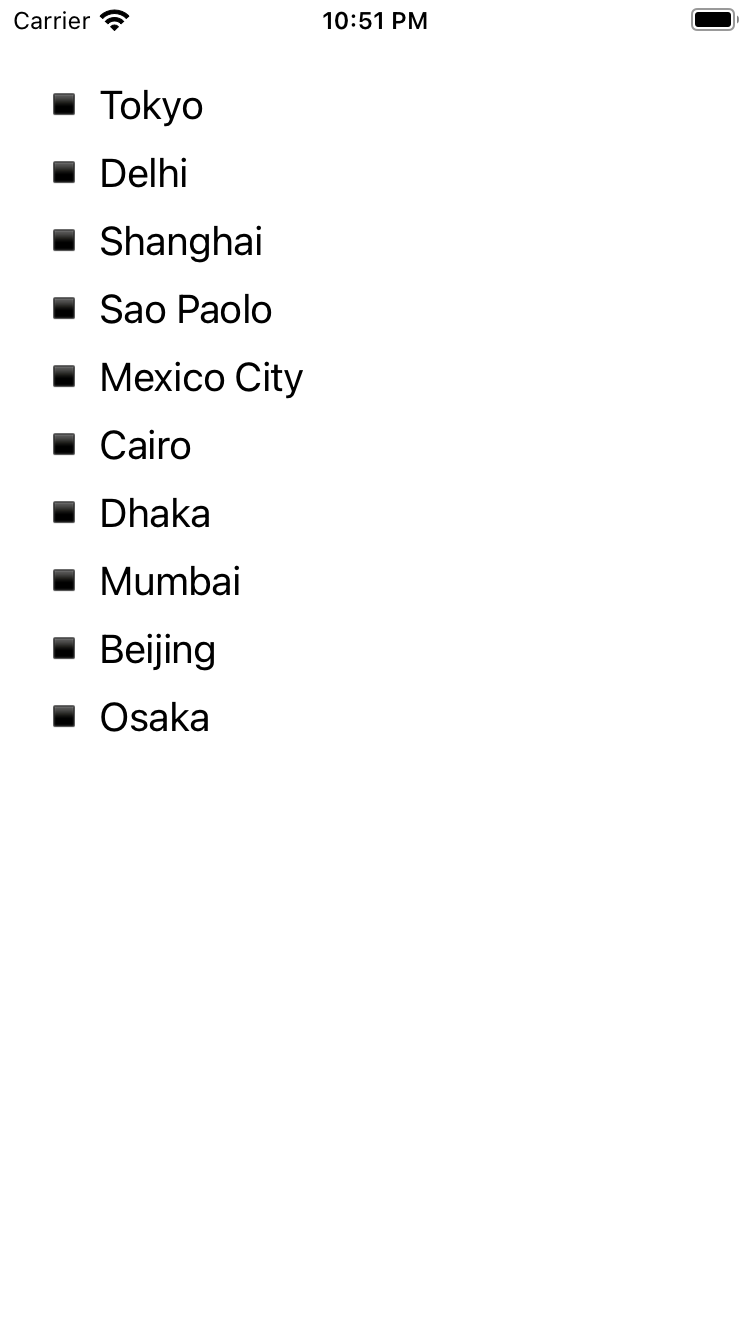
Circled bullet
You can use a circled bullet by using the u29BF unicode:
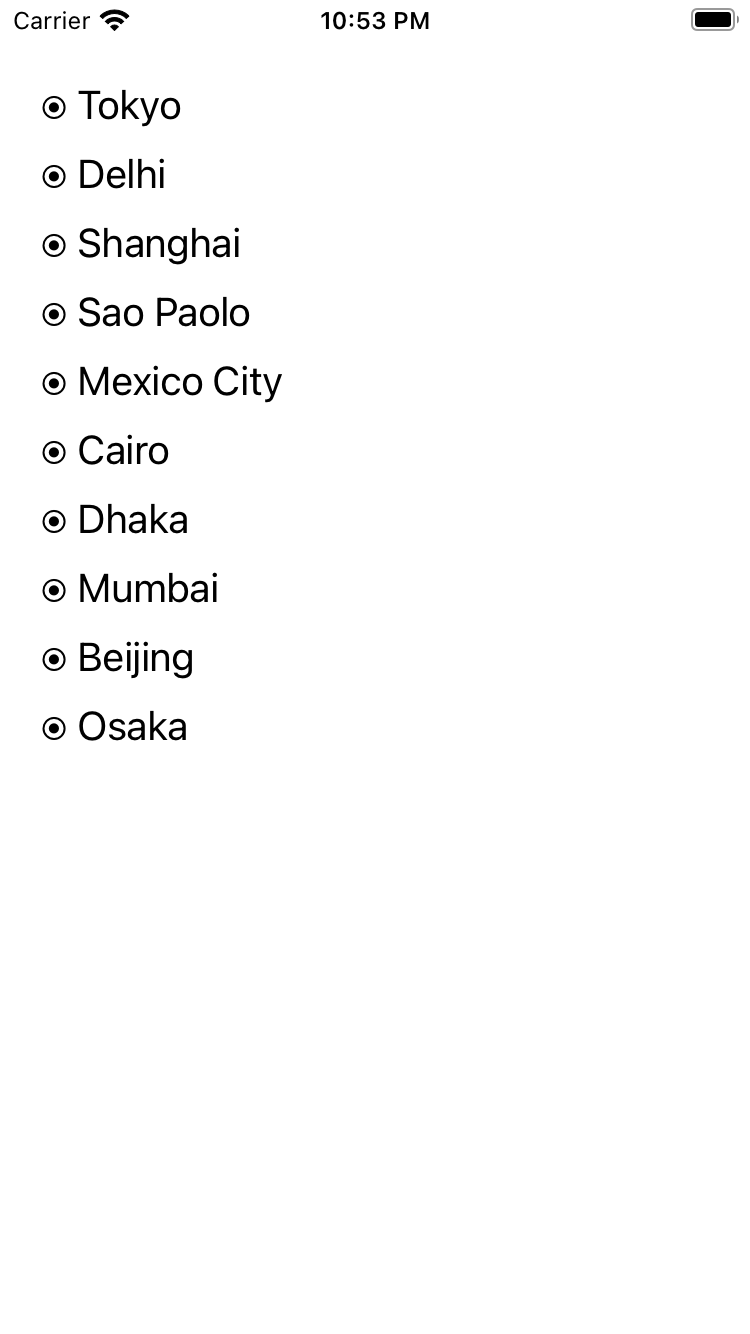
Triangle bullet
You can use a hyphenated bullet by using the u2023 unicode:
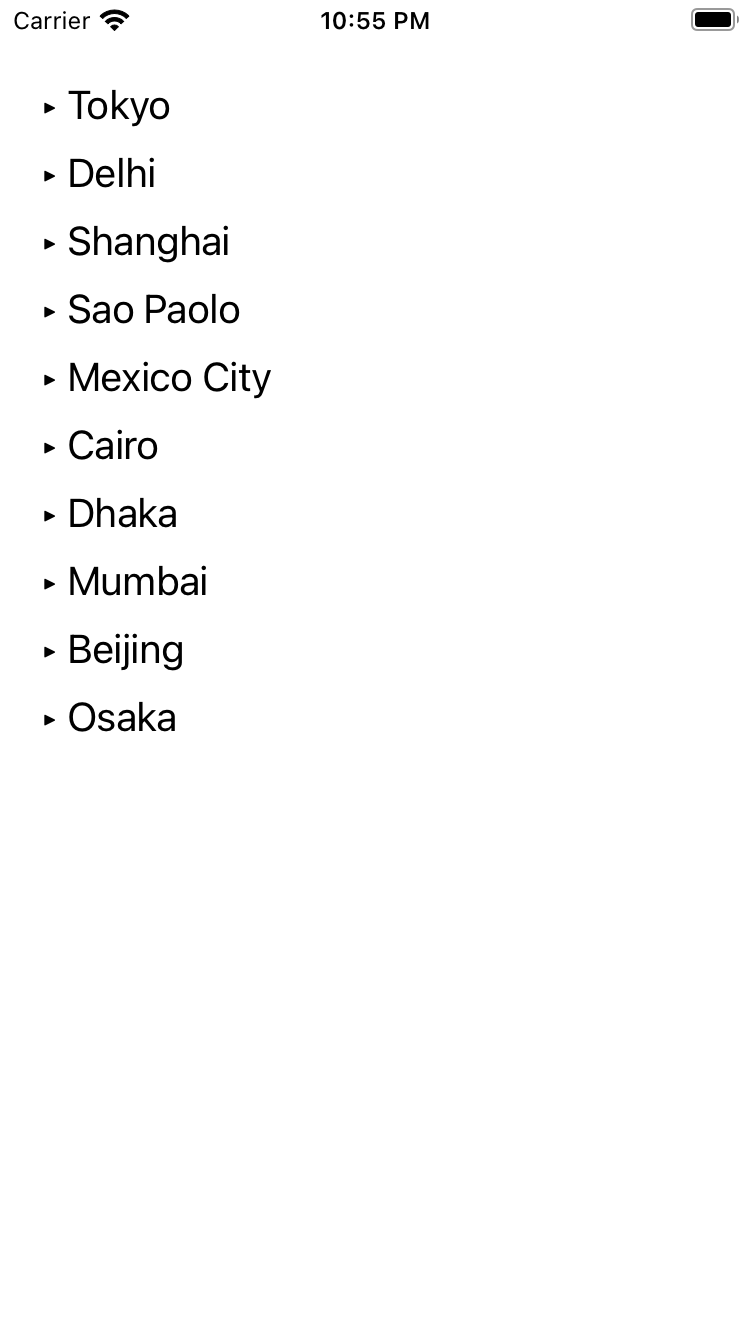